How to Send HTTP Headers With cURL
TLDR: key takeaways
- cURL is a powerful command-line tool for making HTTP requests and customizing headers
- Use the -H or --header option to set or modify HTTP headers in your cURL requests
- You can alter default cURL headers like User-Agent and Accept or send completely custom headers for specific needs
If you've ever needed to test an API, debug a web request, or automate interactions with a website, chances are you've used cURL. This versatile command-line tool lets you send HTTP requests with ease. One of cURL's most useful features is the ability to customize the HTTP headers sent with each request. In this guide, we'll explore how to harness the power of cURL headers to gain more control over your HTTP communication.
Introduction to cURL and HTTP Headers
What is cURL?
cURL (client URL) is an open-source command-line tool and library for transferring data using various network protocols. It supports HTTP, HTTPS, FTP, FTPS, and many more. cURL is widely used for testing APIs, debugging network issues, and automating web requests.
What are HTTP Headers?
HTTP headers are key-value pairs sent with an HTTP request or response. They provide additional information about the request or response, such as the content type, encoding, cache settings, and more. Headers are an essential part of the HTTP protocol and allow the client and server to exchange metadata.
Why Customize HTTP Headers?
There are many reasons you might want to customize the HTTP headers in your cURL requests:
- To set the desired response format (e.g., JSON or XML)
- To provide authentication credentials
- To specify caching preferences
- To mimic a particular user agent or browser
- To include custom metadata for the server to process
Sending HTTP Headers with cURL
The -H or --header Option
To set an HTTP header in your cURL request, use the -H
or --header
option followed by the header name and value. For example:
curl -H "Content-Type: application/json" https://api.example.com/data
Syntax for Setting Headers
The general syntax for setting a header with cURL is:
curl -H "Header-Name: Header-Value" URL
Replace Header-Name
with the name of the header field, and Header-Value
with the desired value. Make sure there's a colon and space between the name and value.
Examples of Common Headers to Set
Here are some common HTTP headers you might set with cURL:
- Content-Type: Specifies the media type of the request body (e.g.,
application/json
,application/xml
) - Accept: Indicates the acceptable response formats (e.g.,
application/json
,text/html
) - Authorization: Provides authentication credentials (e.g.,
Bearer token123
) - User-Agent: Identifies the client software making the request
- Referer: Specifies the URL of the previous web page from which a link to the currently requested page was followed
For instance, to set the Accept
header to request JSON responses:
curl -H "Accept: application/json" https://api.example.com/users
Modifying Default cURL Headers
Changing the User-Agent Header
By default, cURL sets the User-Agent
header to something like curl/7.68.0
. Some servers may block requests with unfamiliar user agents. To change it:
curl -H "User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:93.0) Gecko/20100101 Firefox/93.0" https://example.com
Modifying the Accept Header
Similarly, you can override the default Accept
header if you want to fetch a different content type than cURL's default:
curl -H "Accept: application/xml" https://api.example.com/users
Overriding the Host Header
In some cases, you may need to set the Host
header to a different value than the actual hostname in the URL. To override it:
curl -H "Host: test.example.com" https://example.com
Sending Custom HTTP Headers
Creating Your Own Header Fields
In addition to standard headers, you can create and send your own custom HTTP headers. This can be useful for providing application-specific metadata or instructions to the server. To send a custom header, simply provide the desired name and value:
curl -H "X-Custom-Header: foo123" https://example.com
Use Cases for Custom Headers
Custom headers have numerous potential applications, such as:
- Implementing custom authentication schemes
- Passing additional parameters or settings to an API
- Providing contextual data for logging or analytics
- Controlling caching behavior on a per-request basis
Limitations and Considerations
When creating custom headers, keep the following in mind:
- Header names are case-insensitive
- Header names should not contain spaces, newlines, or colons
- Some servers may ignore or strip unrecognized headers
- Very long headers may be rejected by servers
Advanced cURL Header Techniques
Sending Multiple Headers
To set multiple headers in a single cURL command, simply repeat the -H
option for each header:
curl -H "Header1: Value1" -H "Header2: Value2" -H "Header3: Value3" https://example.com
Removing Headers
If you want to remove a header that cURL sets by default, set it to an empty value:
curl -H "User-Agent:" https://example.com
The colon after the header name with no value tells cURL to omit that header from the request entirely.
Inspecting Response Headers
To view the HTTP headers returned by the server, use the -i
or --include
option:
curl -i https://example.com
This will display the response headers as well as the response body in cURL's output. You can also use -I
or --head
to fetch only the headers without the body.
Our process is simple and easy.
Get your proxy in 60 seconds.
For most of our proxy packages we have automated system that will create an account and issue a new proxy for you. Typically we process your order under 60 seconds. Yes, this is that easy and fast.
Select proxy package
Make a payment
Get proxy details in your panel and email
Troubleshooting Header Issues
Common Errors and Solutions
- 400 Bad Request: Malformed header syntax. Check for typos, incorrect spacing, or invalid characters.
- 403 Forbidden: The server blocked the request. Ensure you have the proper permissions and are setting any required authentication headers.
- 406 Not Acceptable: The server can't return a response matching your Accept header. Verify the header value is correct.
Debugging with Verbose Mode
If you're having trouble with headers, cURL's verbose mode can be a valuable debugging tool. Add the -v
or --verbose
flag to your command:
curl -v -H "X-Debug: true" https://example.com
This will print detailed information about the request and response, including all headers sent and received.
Online Tools for Testing Headers
There are also several web-based tools that can help you test and debug HTTP headers:
- RequestBin - Lets you inspect requests made to a unique URL, including headers
- httpbin - Provides endpoints for testing various HTTP scenarios
- Webhook.site - Similar to RequestBin, generates custom URLs to capture and inspect requests
Conclusion and Further Reading
HTTP headers provide a powerful mechanism for controlling and customizing web requests. By leveraging cURL's header options, you can set default headers, add your own custom fields, and debug header-related issues. Headers are an indispensable tool for anyone working with web APIs or HTTP in general.
To learn more about cURL and HTTP headers, check out these resources:
- The cURL project - Official documentation
- HTTP headers (Mozilla Developer Network) - Comprehensive guide to HTTP headers
- Header Field Definitions (W3C) - Formal definitions of standard headers
Using the appropriate HTTP headers in combination with the features of POST and GET requests will allow you to effectively communicate with web servers and APIs. Pairing cURL with high-quality litport.net proxies can further enhance your ability to make reliable requests.
Frequently Asked Questions
Can I send multiple values for the same header in cURL?
Yes, to send multiple values for a single header field, separate them with commas:
curl -H "Accept: application/json, text/plain" https://example.com
Is there a limit to the number of headers I can send?
cURL itself doesn't limit the number of headers. However, servers may impose their own limits on the total number or size of headers they accept in a request. Sending an excessive number of headers may result in a 400 Bad Request error.
What happens if I set the same header multiple times?
If you specify the same header multiple times with different values, cURL will send all the specified values for that header field. The order in which the values appear in the request is the same as the order of the -H
options in your command.
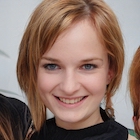