HTTP 412 Precondition Failed: A Developer's Guide to Prevention and Resolution
Key Takeaways
- HTTP 412 occurs when request preconditions (like ETags or timestamps) don't match server expectations
- Common triggers include outdated caches, concurrent modifications, and mismatched conditional headers
- Prevention strategies include proper ETag implementation, atomic operations, and robust error handling
- Modern frameworks and CDNs provide built-in tools to handle conditional requests effectively
- Regular monitoring and testing are crucial for early detection and prevention
Understanding HTTP 412 Errors
The HTTP 412 Precondition Failed status code is a client error response indicating that one or more conditions in the request header fields failed validation. This error commonly occurs in modern web applications, especially those handling concurrent updates or implementing caching mechanisms. Understanding this status code is crucial for developers building robust distributed systems, as it helps prevent data inconsistencies and race conditions that could compromise application integrity. The 412 status code is part of the HTTP/1.1 specification and serves as a safeguard mechanism for conditional operations. When a client makes a request with specific preconditions (using headers like If-Match, If-None-Match, If-Modified-Since, or If-Unmodified-Since), the server evaluates these conditions before processing the request. If any condition fails, the server responds with a 412 status code, effectively preventing potentially harmful operations from being executed. For a comprehensive overview of HTTP error codes and their solutions, check out our complete guide to proxy error codes.
Why HTTP 412 Matters
In today's distributed systems, maintaining data consistency while handling multiple concurrent requests is crucial. The HTTP 412 status code plays a vital role in preventing race conditions and ensuring data integrity. This becomes particularly important in microservices architectures, where multiple services might attempt to modify the same resource simultaneously, or in scenarios with high-frequency API calls where timing issues could lead to data corruption. The implementation of proper precondition handling using HTTP 412 responses can prevent many common distributed systems problems, such as the lost update problem, where one user's changes might inadvertently overwrite another's. By leveraging conditional requests and proper status code handling, applications can maintain ACID properties (Atomicity, Consistency, Isolation, Durability) even in highly concurrent environments.
Common Causes of HTTP 412 Errors
1. ETag Mismatches
ETags are unique identifiers that represent specific versions of a resource. When a client includes an If-Match or If-None-Match header with an outdated ETag, the server returns a 412 error. This commonly occurs in:
- Concurrent edit scenarios
- Cached resources that have been modified
- Distributed systems with replication lag
2. Timestamp-Related Issues
Headers like If-Modified-Since and If-Unmodified-Since can trigger 412 errors when:
- Client and server clocks are not synchronized
- Caching layers introduce timestamp discrepancies
- Resources are modified between requests
3. Conditional Request Problems
Modern web applications use conditional requests for:
- Optimistic concurrency control
- Cache validation
- Resource state verification
Prevention Strategies
1. Implement Proper ETag Handling
// Example Node.js ETag implementation const generateETag = (resource) => { const hash = crypto .createHash('sha256') .update(JSON.stringify(resource)) .digest('hex'); return `"${hash}"`; }; app.get('/api/resource/:id', (req, res) => { const resource = getResource(req.params.id); const etag = generateETag(resource); if (req.header('If-None-Match') === etag) { return res.status(304).send(); } res.setHeader('ETag', etag); res.json(resource); });
2. Use Atomic Operations
When possible, implement atomic operations to prevent race conditions:
// Example using MongoDB's findOneAndUpdate const updateResource = async (id, update, version) => { const result = await collection.findOneAndUpdate( { _id: id, version: version // Optimistic concurrency control }, { $set: update, $inc: { version: 1 } }, { returnDocument: 'after' } ); if (!result.value) { throw new Error('412 Precondition Failed'); } return result.value; };
3. Implement Robust Error Handling
Create a standardized error handling approach:
class PreconditionFailedError extends Error { constructor(message) { super(message); this.name = 'PreconditionFailedError'; this.statusCode = 412; } } // Error handling middleware app.use((err, req, res, next) => { if (err instanceof PreconditionFailedError) { return res.status(412).json({ error: 'Precondition Failed', message: err.message, retryAfter: 5 // Suggest retry interval }); } next(err); });
Modern Tools and Solutions
Content Delivery Networks (CDNs)
Modern CDNs provide built-in support for conditional requests. For example, Cloudflare automatically handles ETag generation and validation, reducing the burden on origin servers. Learn more about avoiding blocks while making requests in our guide on how to scrape websites without getting blocked.
Monitoring and Testing
1. Implementation Testing
Create comprehensive tests for conditional request scenarios:
describe('Resource API', () => { it('should return 412 when ETag mismatches', async () => { const resource = await createResource(); const oldEtag = resource.etag; await updateResource(resource.id); const response = await client.put(`/api/resources/${resource.id}`, { headers: { 'If-Match': oldEtag } }); expect(response.status).toBe(412); }); });
2. Monitoring Strategy
Implement monitoring for 412 errors:
- Set up alerts for unusual spikes in 412 responses
- Track error patterns by endpoint and client
- Monitor response times for conditional requests
Community Perspectives
Developer discussions across technical forums reveal significant challenges when dealing with HTTP 412 errors in production environments. Recent experiences shared by engineers highlight both the complexity of handling these errors and innovative approaches to resolution. To learn more about preventing proxy blocks and errors, check out our guide on how to reduce the risk of getting proxies blocked.
Real-World Implementation Stories
A notable case study from January 2025 documents widespread HTTP 412 and 407 errors affecting Samsung device users on the Verizon network during a software update rollout. Technical teams encountered several recurring patterns:
- Update failures consistently occurring around 50-60% completion
- Device resource exhaustion during prolonged download attempts
- Varying success rates with alternative update methods
- Hardware-specific dependencies affecting error resolution
Engineers implementing workarounds reported mixed results, with some finding success using manufacturer-provided update tools, while others noted these solutions weren't universally accessible. This scenario particularly highlights the importance of robust error handling and graceful degradation in update systems.
Lessons Learned
The developer community's experiences emphasize several key considerations for preventing and handling HTTP 412 errors:
- Implement distributed update delivery systems with failover capabilities
- Design progressive download mechanisms that can resume from failure points
- Provide clear user communication about error states and recovery options
- Ensure multiple update paths for different user scenarios
- Consider hardware and network constraints in error handling strategies
These real-world insights demonstrate how HTTP 412 errors in critical infrastructure can cascade into broader user experience issues, reinforcing the need for comprehensive error handling strategies.
Future Considerations
Emerging Standards and Best Practices
The HTTP Working Group is actively developing new standards and best practices for handling conditional requests in modern web applications. These developments are particularly focused on addressing the challenges of highly distributed systems and edge computing environments. The upcoming changes and considerations include:
HTTP/3 Improvements
The HTTP Working Group is considering new conditional request mechanisms for HTTP/3, including:
- Enhanced cache validation
- Improved concurrency control
- Better support for distributed systems
Security Considerations
When implementing HTTP 412 handling mechanisms, it's crucial to consider security implications:
- Avoid exposing sensitive information in error messages or response headers
- Implement proper authentication and authorization checks before processing conditional requests
- Use secure hash functions for generating ETags to prevent tampering
- Consider rate limiting and request validation to prevent denial of service attacks
- Maintain audit logs of precondition failures for security analysis
Performance Impact
Proper implementation of HTTP 412 handling can significantly impact application performance:
- Reduced network bandwidth through effective cache validation
- Decreased server load by preventing unnecessary operations
- Improved response times through optimistic concurrency control
- Better resource utilization with proper conditional request handling
Conclusion
HTTP 412 errors, while sometimes challenging, are an essential mechanism for maintaining data consistency in web applications. By implementing proper ETag handling, using atomic operations, and leveraging modern tools, developers can effectively prevent and handle these errors. Regular monitoring and testing ensure that conditional request handling remains robust as applications evolve. Remember that proper handling of HTTP 412 errors is not just about error prevention—it's about building reliable, scalable web applications that can handle concurrent operations while maintaining data integrity. The investment in proper precondition handling pays dividends in application reliability, data consistency, and user experience. As web applications continue to grow in complexity and scale, the importance of proper HTTP 412 handling becomes increasingly critical. By following the guidelines and best practices outlined in this guide, developers can build more robust and reliable distributed systems that can handle the challenges of modern web development.
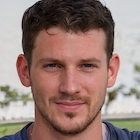