JavaScript Heap Memory: A Developer's Guide to Performance Optimization
Key Takeaways
- JavaScript heap memory stores objects, arrays, and other complex data structures, while primitive values are stored on the stack.
- Common causes of memory leaks include forgotten event listeners, accidental closures, global variables, and detached DOM elements.
- To fix "JavaScript heap out of memory" errors, increase allocated memory using the NODE_OPTIONS environment variable with --max-old-space-size flag.
- Modern memory optimization techniques include using WeakMap/WeakSet, implementing batch processing, and leveraging the FinalizationRegistry API.
- Regular memory profiling using Chrome DevTools or Node.js profiling tools is essential for maintaining healthy application performance.
Understanding JavaScript Heap Memory: The Foundation
When your JavaScript application crashes with a "JavaScript heap out of memory" error, it's not just frustrating—it can bring your entire application to a halt, disappoint users, and in production environments, potentially cost your business money. To build robust applications, you need to understand what happens under the hood.
What is Heap Memory?
JavaScript manages memory in two primary locations:
Stack Memory | Heap Memory |
---|---|
Stores primitive values (numbers, booleans, etc.) | Stores objects, arrays, functions, and other complex data |
Fixed size, fast access (LIFO) | Dynamic allocation, accessed via references |
Automatically cleaned when execution leaves scope | Managed by garbage collection |
Think of heap memory as a dynamic warehouse where JavaScript stores "boxes" (objects) of different sizes. Each time you create an object, array, or function, JavaScript allocates space in this warehouse. The heap has limits, and when you try to store more than it can handle, you encounter that dreaded "out of memory" error.
The JavaScript Memory Lifecycle
Memory in JavaScript follows a three-stage lifecycle:
- Allocation: Memory is reserved when you create variables, objects, or functions
- Usage: The allocated memory is read from or written to during program execution
- Release: Memory is freed when it's no longer needed (handled by garbage collection)
Unlike languages like C++ or Rust where developers manually manage memory, JavaScript handles this automatically through garbage collection—a double-edged sword that makes development easier but can hide memory issues until they become serious problems.
Common Causes of JavaScript Memory Leaks
Memory leaks occur when memory that's no longer needed isn't released. Here are the most common culprits:
1. Forgotten Event Listeners
Event listeners maintain references to the variables in their scope. If not properly removed, they prevent garbage collection:
function setupHandler() { const heavyData = new Array(1000000).fill('🐘'); document.getElementById('button').addEventListener('click', () => { console.log(heavyData.length); }); } // This gets called multiple times without cleanup setInterval(setupHandler, 1000);
The solution is to always remove event listeners when they're no longer needed:
function setupHandler() { const heavyData = new Array(1000000).fill('🐘'); const handler = () => { console.log(heavyData.length); }; const button = document.getElementById('button'); button.addEventListener('click', handler); // Return cleanup function return () => { button.removeEventListener('click', handler); }; } let cleanup; setInterval(() => { // Clean up previous handler before setting up new one if (cleanup) cleanup(); cleanup = setupHandler(); }, 1000);
2. Accidental Closures
Closures can inadvertently keep large objects in memory:
function processData() { const hugeData = new Array(10000000).fill('🐘'); return { getDataSize: () => hugeData.length, // hugeData is kept in memory even though we only need its length }; } const dataProcessor = processData();
A better approach is to extract only what you need:
function processData() { const hugeData = new Array(10000000).fill('🐘'); const size = hugeData.length; return { getDataSize: () => size, // Now hugeData can be garbage collected }; }
3. Global Variables
Variables declared in the global scope persist for the lifetime of your application:
// ❌ Bad practice var globalCache = {}; function processItem(item) { globalCache[item.id] = item; // This cache will grow unbounded } // ✅ Better: scope the cache and implement cleanup function createProcessor() { const cache = {}; return { process: (item) => { cache[item.id] = item; }, clearCache: () => { for (const key in cache) { delete cache[key]; } } }; }
4. Detached DOM Elements
A particularly insidious form of memory leak occurs when you remove DOM elements from the document but keep JavaScript references to them:
// Store reference to element const element = document.getElementById('myElement'); // Remove from DOM element.parentNode.removeChild(element); // But the JavaScript reference still exists! // element still consumes memory
The solution is to nullify the reference after removal:
const element = document.getElementById('myElement'); element.parentNode.removeChild(element); element = null; // Now it can be garbage collected
How Garbage Collection Works in JavaScript
JavaScript engines use automatic garbage collection to reclaim memory no longer in use. Understanding this process helps you write more memory-efficient code.
The Mark-and-Sweep Algorithm
Modern JavaScript engines primarily use a mark-and-sweep algorithm for garbage collection:
- Mark Phase: The garbage collector identifies and marks all objects that are still reachable from the root objects (global objects, currently executing functions)
- Sweep Phase: All unmarked objects (unreachable) are removed, and their memory is freed
This process solves the circular reference problem that plagued older reference-counting systems:
function createCircularReference() { let obj1 = {}; let obj2 = {}; // Create circular reference obj1.ref = obj2; obj2.ref = obj1; // Both objects will be garbage collected // when this function completes }
Memory Allocation vs. Garbage Collection: Finding Balance
JavaScript's garbage collection process isn't free—it requires CPU time and can cause noticeable pauses in your application. Mozilla research showed that garbage collection can account for up to 15-20% of execution time in complex web applications.
Finding the balance between memory usage and garbage collection frequency is crucial for performance-sensitive applications.
Fixing "JavaScript Heap Out of Memory" Errors
The most immediate solution to heap out of memory errors is increasing the allocated memory:
For Node.js Applications
You can increase the memory limit by setting the NODE_OPTIONS environment variable:
On Windows:
// In PowerShell (temporary) $env:NODE_OPTIONS="--max-old-space-size=4096" // Or create a permanent environment variable: // NODE_OPTIONS=--max-old-space-size=4096
On macOS/Linux:
// In terminal (temporary) export NODE_OPTIONS=--max-old-space-size=4096 // Or add to your shell profile file (.bashrc, .zshrc, etc.)
The value should be in megabytes (MB), so 4096 allocates 4GB of memory. Choose a value appropriate for your system—allocating too much can cause system instability.
For Browser Applications
Browser memory limits can't be directly controlled, but you can optimize your web application:
- Split large processes into smaller chunks with setTimeout/requestAnimationFrame
- Implement virtual scrolling for large lists
- Use web workers for memory-intensive operations
- Consider using rotating proxies for distributing load when handling large-scale data operations
Advanced Memory Management Techniques
While increasing memory limits provides immediate relief, implementing proper memory management techniques offers long-term benefits:
1. Use Weak References
ES6 introduced WeakMap and WeakSet, which allow keys to be garbage collected when no other references exist:
// ❌ Regular Map keeps references even if objects are no longer used const cache = new Map(); // ✅ WeakMap allows objects to be garbage collected const cache = new WeakMap(); function processUser(user) { if (cache.has(user)) { return cache.get(user); } const result = expensiveOperation(user); cache.set(user, result); return result; }
2. Implement Batch Processing
When dealing with large datasets, process them in smaller batches to allow garbage collection between operations:
async function processBatch(items, batchSize = 1000) { for (let i = 0; i < items.length; i += batchSize) { const batch = items.slice(i, i + batchSize); await Promise.all(batch.map(processItem)); // Allow garbage collection between batches await new Promise(resolve => setTimeout(resolve, 0)); } }
3. Stream Large Data
Instead of loading entire files into memory, use streams:
// ❌ Don't load entire files into memory const fs = require('fs'); fs.readFile('large-file.csv', (err, data) => { const lines = data.toString().split('\n'); lines.forEach(processLine); }); // ✅ Process data as it comes in const readline = require('readline'); const fs = require('fs'); const fileStream = fs.createReadStream('large-file.csv'); const rl = readline.createInterface({ input: fileStream, crlfDelay: Infinity }); rl.on('line', (line) => { processLine(line); });
This approach is especially important when scraping websites or processing large datasets, where memory constraints can lead to failures.
4. Use Object Pooling for Frequent Allocations
Object pooling reuses objects instead of creating new ones, reducing garbage collection overhead:
class ObjectPool { constructor(factory, initialSize = 10) { this.factory = factory; this.pool = Array(initialSize).fill().map(() => factory()); } acquire() { if (this.pool.length > 0) { return this.pool.pop(); } return this.factory(); } release(obj) { this.pool.push(obj); } } // Usage const vectorPool = new ObjectPool(() => ({ x: 0, y: 0 })); function processVectors() { const vec = vectorPool.acquire(); vec.x = 10; vec.y = 20; // Use vector... // Reset and return to pool vec.x = 0; vec.y = 0; vectorPool.release(vec); }
5. Use the FinalizationRegistry API
The FinalizationRegistry API (introduced in ES2021) allows you to register callbacks that execute when objects are garbage collected, useful for cleanup operations and monitoring:
const registry = new FinalizationRegistry((key) => { console.log(`Object with key ${key} has been garbage collected.`); }); function createLargeObject() { const obj = { data: new Array(1000000).fill('data'), performCleanup: () => { /* cleanup logic */ } }; // Register object with the registry registry.register(obj, "largeObject"); return obj; } let obj = createLargeObject(); // Later, when we're done obj = null; // When garbage collection runs, our callback will execute
Monitoring and Profiling Memory Usage
Proactive monitoring helps identify memory issues before they become critical. This is particularly important for applications that handle data scraping operations or process large datasets.
Using Chrome DevTools
Chrome DevTools provides powerful memory analysis capabilities:
- Open DevTools (F12 or Ctrl+Shift+I)
- Go to the Memory tab
- Take heap snapshots at different points in your application lifecycle
- Compare snapshots to identify memory growth
- Look for detached DOM elements and unexpected object retention
Recent updates to Chrome DevTools in 2024 include improved allocation profiling and memory usage visualization, making it easier to identify problematic patterns.
Node.js Memory Profiling
For Node.js applications, implement basic memory monitoring:
function logMemoryUsage() { const used = process.memoryUsage(); console.log({ heapTotal: `${Math.round(used.heapTotal / 1024 / 1024)} MB`, heapUsed: `${Math.round(used.heapUsed / 1024 / 1024)} MB`, external: `${Math.round(used.external / 1024 / 1024)} MB`, rss: `${Math.round(used.rss / 1024 / 1024)} MB` }); } // Log memory usage periodically setInterval(logMemoryUsage, 30000);
For more comprehensive analysis, consider tools like Clinic.js and Node.js diagnostic tooling.
Memory Monitoring Alert System
Implement an alert system to catch memory issues early:
class MemoryMonitor { constructor(threshold = 0.9, alertCallback) { this.threshold = threshold; this.lastUsage = 0; this.alertCallback = alertCallback || console.warn; } check() { const { heapUsed, heapTotal } = process.memoryUsage(); const usage = heapUsed / heapTotal; if (usage > this.threshold) { this.alertCallback(`Memory usage critical: ${Math.round(usage * 100)}%`); } // Track memory growth const growth = heapUsed - this.lastUsage; if (growth > 100 * 1024 * 1024) { // 100MB growth this.alertCallback(`Significant memory growth: ${Math.round(growth / 1024 / 1024)}MB`); } this.lastUsage = heapUsed; } } const monitor = new MemoryMonitor(0.85, (msg) => { // Send to logging service or alert system console.warn(msg); }); setInterval(() => monitor.check(), 60000);
Case Study: Memory Optimization in a Real-World Application
In 2023, a major e-commerce platform was experiencing significant performance degradation during peak traffic periods. Analysis revealed their product catalog browser—displaying thousands of items with complex filtering—was leaking memory.
The primary issues identified were:
- Event listeners on filter components weren't being properly removed when filters changed
- Product data was being cached globally without size limitations
- DOM elements for products scrolled out of view weren't being recycled
The team implemented a three-pronged approach:
- Switched to a delegated event model with a single listener on the container
- Implemented an LRU cache with WeakMap for product data
- Adopted virtual scrolling to maintain only visible DOM elements
The results were impressive:
- Memory usage reduced by 64%
- Page responsiveness improved by 42%
- Server infrastructure costs decreased by 28% due to improved client-side performance
The Future of JavaScript Memory Management
JavaScript runtime environments continue to evolve with better memory management capabilities:
Recent Developments
- Incremental Garbage Collection: Reduces pause times by breaking collection into smaller steps
- Concurrent Garbage Collection: Allows collection to run alongside application code
- Memory Instrumentation APIs: Provide developers with deeper insights into memory usage patterns
According to the V8 team's blog, the latest garbage collection optimizations have reduced collection pause times by up to 70% in typical web applications.
Emerging Best Practices
As JavaScript continues to power more complex applications, these best practices are becoming increasingly important:
- Memory Budgeting: Establish performance budgets for memory usage and monitor regularly
- Modular Architecture: Design systems that load only what's needed and unload modules when not in use
- Automated Memory Testing: Integrate memory usage tests into CI/CD pipelines
Community Perspectives: Real-World Experiences
Technical discussions across various platforms reveal that JavaScript memory management—especially dealing with heap memory limits—is a common pain point for developers working on complex projects. The conversations highlight both practical solutions and underlying frustrations with memory constraints in modern web development environments.
Angular developers in particular have shared their struggles with memory limitations during build processes. One developer reported consuming all 16GB of RAM on their system during an Angular build, even with relatively common tooling running alongside. The most common recommendation from the community is increasing Node.js memory allocation using the --max-old-space-size
flag, typically implemented by adding custom npm scripts in package.json ("build": "node --max-old-space-size=4096 node_modules/@angular/cli/bin/ng build"
) or setting environment variables.
Beyond configuration adjustments, engineers have identified deeper architectural issues contributing to memory problems. Many point to project bloat with excessive third-party dependencies as a significant factor, while others note that certain development environments like WebStorm may exacerbate the issue. Some developers report that system-level solutions like adding swap files in Linux environments can prevent crashes, suggesting that memory management extends beyond the application level.
Technical teams have also discovered how asynchronous programming patterns significantly impact memory consumption, particularly when handling large datasets. A common mistake identified in community discussions is creating too many simultaneous Promises with map()
without properly awaiting their resolution through Promise.all()
, effectively creating a self-inflicted denial-of-service situation. For database operations with large datasets, experienced developers often recommend batch processing, implementing manual for-loops with await, or using specialized tools instead of Node.js for certain operations. These are among the common mistakes beginners make when working with data-intensive applications.
While increased memory allocation provides immediate relief, the community generally acknowledges this as a temporary solution rather than addressing root causes. The conversations reveal a pragmatic developer community that balances quick fixes for immediate productivity against the recognition that optimizing code architecture, managing dependencies carefully, and understanding asynchronous patterns are equally important for sustainable memory management.
Conclusion: Balancing Performance and Resource Usage
JavaScript memory management is a balancing act. While the language's automatic garbage collection simplifies development, it doesn't eliminate the need for thoughtful memory management. By understanding how the heap works, identifying common causes of memory leaks, and implementing proper monitoring and optimization techniques, you can build applications that are both powerful and resource-efficient.
Remember that memory optimization isn't about implementing every technique possible—it's about identifying the specific bottlenecks in your application and addressing them with the appropriate solutions. Start with monitoring, measure before optimizing, and always consider the trade-offs between complexity and performance gains.
With the strategies outlined in this guide, you'll be well-equipped to tackle memory challenges in your JavaScript applications, whether they run in the browser or on the server.
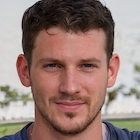