Go vs Python for Web Scraping: The Ultimate Performance-Focused Guide (2025)
Key Takeaways
- Python excels in web scraping with rich libraries and ease of use, making it ideal for beginners and complex parsing.
- Go offers superior performance with faster execution speeds—up to 47% faster than Python for large-scale scraping operations.
- Python takes the lead for quick prototyping and complex websites, while Go shines in enterprise-level, high-volume scraping.
- Recent benchmarks show Go scraping 500M URLs in 343 days versus Python's 649 days.
- The optimal choice depends on specific project requirements: Python for flexibility and libraries, Go for performance and scalability.
Introduction: The Web Scraper's Dilemma
When embarking on a web scraping project, you're immediately faced with a critical decision: which programming language will best serve your needs? Among the contenders, Python and Go consistently emerge as leading options, each with distinct advantages that appeal to different scraping scenarios.
Python has long dominated the web scraping landscape with its user-friendly syntax and extensive ecosystem of specialized libraries. Meanwhile, Go (Golang) has gained significant traction as developers recognize its exceptional performance benefits for large-scale data collection.
This guide dives deep into the Python vs Go debate specifically for web scraping applications, providing you with concrete data, real-world comparisons, and expert insights to help you make the optimal choice for your specific requirements. Whether you're building a simple data collection script or an enterprise-grade scraping infrastructure, understanding the strengths and limitations of each language is crucial for success.
Understanding the Languages: Core Characteristics
Python: The Swiss Army Knife
Python is an interpreted, dynamically-typed language first released in 1991. Its philosophy emphasizes code readability and developer productivity, making it accessible to beginners while remaining powerful enough for advanced applications.
Python's key characteristics include:
- Interpreted execution: Code is executed line-by-line by the interpreter
- Dynamic typing: Variable types are checked at runtime
- Extensive standard library with batteries-included philosophy
- Expressive syntax that prioritizes readability
According to the TIOBE Index for February 2025, Python remains the most popular programming language globally, with widespread adoption across various domains including web scraping, data science, and artificial intelligence.
Go: The Performance Powerhouse
Go (or Golang) is a compiled, statically-typed language developed by Google in 2009. It was designed with modern computing infrastructure in mind, focusing on simplicity, efficiency, and built-in support for concurrent programming.
Go's defining features include:
- Compiled execution: Code is translated to machine code before running
- Static typing: Variable types are verified at compile time
- Goroutines: Lightweight threads for efficient concurrency
- Strong memory management with garbage collection
Go has seen a 12% increase in adoption specifically for backend and system programming tasks, including web scraping applications.
Head-to-Head Comparison for Web Scraping
Performance Benchmarks
Performance is often the decisive factor when choosing between Python and Go for web scraping at scale. Recent benchmarks reveal significant differences:
Metric | Python | Go |
---|---|---|
Time to scrape 500M URLs | 649 days | 343 days (47% faster) |
Memory usage per 1000 concurrent requests | ~780MB | ~320MB |
CPU utilization for parsing 1GB HTML | High | Medium |
These performance differences stem from fundamental language characteristics:
- Go's compilation advantage: Pre-compiled machine code executes faster than Python's interpreted bytecode
- Go's concurrency model: Goroutines require less memory overhead than Python threads
- Go's static typing: Enables compiler optimizations that improve memory efficiency
According to research published by ACM Digital Library, Go outperforms Python by 30-50% in network-bound operations typical in web scraping workflows.
Memory Management and Resource Utilization
Web scraping at scale can be resource-intensive, making efficient memory management crucial.
Go offers superior memory efficiency through:
- Static typing that allocates memory precisely
- Efficient garbage collection designed for low latency
- Stack allocation for many variables instead of heap allocation
Python's memory management is less efficient for several reasons:
- Dynamic typing requires additional memory for type information
- Reference counting and cycle detection in garbage collection
- Global Interpreter Lock (GIL) limiting true parallel execution
Library Ecosystem and Development Speed
The availability of specialized libraries directly impacts development speed and capability.
Python's Rich Ecosystem
Python offers a mature ecosystem for web scraping:
- BeautifulSoup: Simple HTML/XML parsing with intuitive navigation
- Scrapy: Comprehensive framework with built-in features like request queueing
- Selenium/Playwright: Full browser automation for JavaScript-heavy sites
- Pandas: Powerful data manipulation and analysis
- LXML: Fast XML/HTML processing with XPath support
According to PyPI Stats, BeautifulSoup alone averages over 15 million weekly downloads as of January 2025, demonstrating Python's dominance in the web scraping space.
Go's Growing Arsenal
Go's ecosystem, while smaller, contains powerful tools:
- Colly: Fast and elegant scraping framework with concurrent request handling
- Goquery: jQuery-like HTML parsing and manipulation
- Chromedp: Chrome DevTools Protocol implementation for headless browsing
- Rod: High-level Chrome DevTools Protocol driver
Despite having fewer libraries, Go's standard library is comprehensive, providing robust HTTP handling and HTML parsing capabilities out of the box.
Code Complexity and Readability
The verbosity and readability of code directly impact development and maintenance costs.
Let's compare equivalent web scraping code in both languages:
Python Example (Using Requests + BeautifulSoup)
import requests from bs4 import BeautifulSoup # Send GET request to target page response = requests.get("https://example.com/products") # Parse HTML content soup = BeautifulSoup(response.text, "html.parser") # Find all product elements products = soup.find_all("div", class_="product") # Extract data from each product for product in products: name = product.find("h2").text.strip() price = product.find("span", class_="price").text.strip() print(f"Product: {name}, Price: {price}")
Go Example (Using Net/HTTP + Goquery)
package main import ( "fmt" "net/http" "github.com/PuerkitoBio/goquery" ) func main() { // Send GET request to target page resp, err := http.Get("https://example.com/products") if err != nil { panic(err) } defer resp.Body.Close() // Parse HTML content doc, err := goquery.NewDocumentFromReader(resp.Body) if err != nil { panic(err) } // Find all product elements and extract data doc.Find("div.product").Each(func(i int, s *goquery.Selection) { name := s.Find("h2").Text() price := s.Find("span.price").Text() fmt.Printf("Product: %s, Price: %s\n", name, price) }) }
The Python example contains 8 lines of actual code, while the Go implementation requires 16 lines. Python's syntax is more concise, but Go provides explicit error handling and a clearer execution flow.
Real-World Use Cases: When to Choose Each Language
When Python Excels for Web Scraping
- Rapid prototyping and exploration - Python's concise syntax enables quick iterations and experimentation.
- Complex data extraction patterns - Python's extensive libraries make handling complex HTML structures easier.
- Integration with data science workflows - Direct compatibility with pandas, numpy, and other data analysis tools.
- Limited technical resources - Lower learning curve and wider talent availability.
Case Study: Media Monitoring Platform
A media analytics startup needed to monitor news sources across multiple countries and languages. They chose Python because:
- The project required frequent adaptation to changing website structures
- Complex content extraction needed NLP capabilities readily available in Python
- The team had varied technical backgrounds but could all work with Python
According to the CTO: "Python's flexibility allowed us to rapidly adjust our scrapers as news sites evolved. When we needed to analyze sentiment or categorize content, the seamless integration with NLP libraries was invaluable."
When Go Dominates Web Scraping
- High-volume, production-grade scraping - Go's performance advantages compound at scale.
- Resource-constrained environments - Lower memory footprint means more concurrent scraping per server.
- Distributed scraping architectures - Go's networking capabilities and concurrency model excel in distributed systems.
- Long-running, mission-critical applications - Go's stability and error handling improve reliability.
Case Study: E-commerce Price Monitoring
An e-commerce intelligence company initially built their price monitoring system in Python but migrated to Go after encountering scaling issues. Their results:
- Reduced server count from 24 to 9 instances
- Decreased scraping cycle time from 6 hours to 2 hours
- Improved reliability with error rates dropping from 8% to under 2%
Their Lead Engineer noted: "The migration to Go was challenging, but the performance and stability gains were transformative. Our scraping infrastructure now handles 5x the volume on fewer resources."
Handling Modern Web Scraping Challenges
JavaScript Rendering Capabilities
Modern websites rely heavily on JavaScript to render content dynamically. Both languages offer solutions for this challenge:
Python's JavaScript Handling
- Selenium/Playwright: Full browser automation with comprehensive APIs
- Pyppeteer: Python port of Puppeteer for Chrome automation
- Splash: Lightweight JavaScript rendering service
Python offers more mature and easier-to-use options for JavaScript rendering, with extensive documentation and community support.
Go's JavaScript Handling
- Chromedp: Chrome DevTools Protocol implementation
- Rod: High-level Chrome automation driver
While Go has fewer options, its JavaScript rendering tools often provide better performance and lower resource usage for headless browser automation.
Avoiding Detection and Blocks
Both languages can implement anti-blocking strategies to avoid getting blocked during scraping operations:
Strategy | Python Implementation | Go Implementation |
---|---|---|
Proxy rotation | Easy with requests-proxy-rotation | Requires more custom code |
User-agent rotation | Simple with built-in libraries | Simple with standard library |
Request throttling | Libraries available | Better native timing control |
Fingerprint randomization | More libraries available | Fewer ready solutions |
Making Your Decision: A Framework
To choose the right language for your web scraping project, consider these factors:
- Scale Requirements:
- Small scale (<100k pages/day): Python is likely sufficient
- Large scale (>100k pages/day): Go's performance advantages become significant
- Team Expertise:
- Python has a lower learning curve and wider developer availability
- Go requires more specialized knowledge but produces more maintainable code in large projects
- Project Complexity:
- Complex parsing and data transformation favors Python's rich ecosystem
- High concurrency and networking requirements favor Go
- Integration Requirements:
- Data science workflow integration favors Python
- Microservices architecture integration favors Go
Hybrid Approaches: Getting the Best of Both Worlds
An emerging trend in 2024-2025 is using both languages together:
- Go-powered scraping engine to handle high-volume HTTP requests, concurrency, and basic HTML processing
- Python-based data processing pipeline for complex transformation, analysis, and integration with ML/AI
According to web scraping architect Maria Rodriguez: "We've seen tremendous success with a hybrid approach where Go handles the heavy lifting of fetching and processing at scale, while Python takes care of the complex data transformations and analysis where its ecosystem shines."
Companies like ScrapingHub have reported 40% improved efficiency by adopting this hybrid model for large-scale scraping operations.
From the Trenches: Developer Experiences
Technical discussions across various platforms reveal that the choice between Go and Python for web scraping often depends on specific project requirements and performance needs. Experienced scrapers point out that while Python offers an extensive ecosystem with libraries like Scrapy that provide multithreading frameworks for efficient data collection, Go's native concurrency capabilities through goroutines offer compelling advantages for large-scale operations. Several developers report transitioning between languages as their projects evolved—starting with Python for prototyping and eventually migrating to Go when performance became critical.
The performance debate continues to generate significant interest in developer communities. Some engineers report substantial speed improvements after migrating their scraping projects from Python to Go, with one developer noting execution times nearly twice as fast (850ms vs 1600ms) after compilation. However, more technically versed community members caution against simplistic benchmarks, highlighting that many comparisons fail to account for compile time, SSL negotiation, network latency, and startup costs—all of which can obscure the true performance differences between the languages.
Both languages ultimately have their place in the web scraping ecosystem, with project requirements dictating the optimal choice. Community wisdom suggests using Python for one-off scrapes, specific site targeting, and situations where development speed outweighs runtime performance. For repeated, high-volume scraping across multiple sites, Go's performance advantages become more compelling. Interestingly, several experienced developers advocate a measured approach to scraping regardless of language choice—emphasizing that slower, more considerate scraping is often better practice to avoid overwhelming target servers.
Conclusion: Choose the Right Tool for Your Scraping Job
The Python versus Go debate for web scraping doesn't have a one-size-fits-all answer. Both languages offer compelling advantages for different scraping scenarios:
Choose Python when:
- You need rapid development and prototyping
- Your project involves complex data extraction patterns
- You're integrating with data science workflows
- Your team has mixed technical backgrounds
Choose Go when:
- Performance at scale is critical
- You're operating in resource-constrained environments
- Your architecture is distributed
- Long-term maintenance and stability are priorities
For many organizations, the optimal approach may involve using both languages strategically—leveraging Go's performance for data acquisition and Python's ecosystem for processing and analysis.
As web scraping continues to evolve alongside increasingly complex websites and anti-scraping measures, the tools and techniques we use must similarly advance. Whether you choose Python, Go, or a hybrid approach, success will ultimately come from understanding the unique requirements of your project and selecting the right tool for the job. If you're just starting out, be sure to avoid common web scraping mistakes that can impact your results.
Further Resources
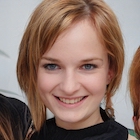