Cloudflare Error 1020: A Developer's Guide to Diagnosis and Solutions
Key Takeaways
- Error 1020 occurs when Cloudflare's anti-bot system detects suspicious activity, typically triggered by automated requests, high-frequency access, or suspicious IP addresses
- For regular users, solutions include clearing browser cache, disabling VPNs, and checking for browser extension conflicts
- Developers can implement rotating proxies, custom User-Agent management, and specialized tools like Undetected ChromeDriver for automated access
- The most reliable solution for large-scale operations is using dedicated scraping APIs that handle Cloudflare bypass automatically
- Always consider legal implications and implement rate limiting to maintain ethical scraping practices
Understanding Cloudflare Error 1020
Cloudflare Error 1020, known as the "Access Denied" error, is a security response triggered when Cloudflare's anti-bot system detects potentially suspicious activity. According to recent data from 2024, this error has become increasingly common, affecting both regular users and developers attempting to access protected websites. For developers interested in scraping websites protected by Cloudflare, understanding these mechanisms is crucial.
What Triggers Error 1020?
Based on analysis of recent cases and Cloudflare's documentation, the error typically occurs due to:
- High-frequency requests from a single IP address (typically more than 50 requests per minute)
- Automated access patterns that deviate from human behavior
- IP addresses associated with known proxy services or VPNs
- Missing or inconsistent browser fingerprints
- Geolocation-based restrictions set by website owners
Solutions for Regular Users
1. Browser-Related Fixes
Start with these browser-specific solutions:
- Clear Browser Data: Remove cookies, cache, and browsing history
- Disable Extensions: Particularly privacy-focused ones that might interfere with Cloudflare's checks
- Update Your Browser: Ensure you're running the latest version with current security protocols
2. Network-Level Solutions
If browser fixes don't work, try these network adjustments. For more details, check our guide on troubleshooting proxy errors:
- Disable VPN: Temporarily turn off VPN services that might trigger Cloudflare's security measures
- Router Reset: Perform a full router restart to obtain a new IP address
- DNS Flush: Clear your DNS cache using the following command:
# For Windows ipconfig /flushdns # For macOS/Linux sudo dscacheutil -flushcache; sudo killall -HUP mDNSResponder
Developer Solutions
1. Implementing Proxy Rotation
A robust proxy rotation system is crucial for maintaining sustained access. Using high-quality residential proxies can significantly improve your success rate. Here's an example using Python's requests library with proxy rotation:
import requests from itertools import cycle proxies = [ {"http": "http://proxy1:port"}, {"http": "http://proxy2:port"}, {"http": "http://proxy3:port"} ] proxy_pool = cycle(proxies) def make_request(url): for i in range(3): # Retry logic try: proxy = next(proxy_pool) response = requests.get( url, proxies=proxy, timeout=30 ) return response except Exception as e: print(f"Error occurred: {e}") continue return None
2. Advanced User-Agent Management
Modern Cloudflare implementations check for consistency between User-Agent strings and other headers. Here's a comprehensive approach:
import random class BrowserProfile: def __init__(self): self.profiles = [ { "user-agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/121.0.0.0 Safari/537.36", "sec-ch-ua": '"Not A(Brand";v="99", "Google Chrome";v="121", "Chromium";v="121"', "sec-ch-ua-platform": '"Windows"', "sec-ch-ua-mobile": "?0" }, # Add more browser profiles ] def get_random_profile(self): return random.choice(self.profiles) def make_request(url): profile = BrowserProfile().get_random_profile() response = requests.get(url, headers=profile) return response
3. Using Undetected ChromeDriver
For scenarios requiring JavaScript execution, Undetected ChromeDriver provides a more reliable solution:
import undetected_chromedriver as uc from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC def scrape_with_undetected_chrome(url): options = uc.ChromeOptions() options.headless = True driver = uc.Chrome(options=options) try: driver.get(url) # Wait for specific element to ensure page load element = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.TAG_NAME, "body")) ) return driver.page_source finally: driver.quit()
Enterprise-Scale Solutions
1. Using Dedicated Scraping APIs
For large-scale operations, dedicated proxy servers for web scraping provide the most reliable solution. They handle proxy rotation, browser fingerprinting, and Cloudflare bypass automatically. Here's an example using a modern scraping API:
import requests def fetch_with_api(url, api_key): endpoint = "https://api.scrapingservice.com/v1/" params = { "api_key": api_key, "url": url, "bypass": "cloudflare_level_1", "js_render": "true" } response = requests.get(endpoint, params=params) return response.json()
2. Implementing Rate Limiting
Proper rate limiting is crucial for maintaining access. Here's a token bucket implementation:
import time from collections import deque class RateLimiter: def __init__(self, tokens_per_second): self.tokens_per_second = tokens_per_second self.tokens = tokens_per_second self.last_update = time.time() self.requests = deque() def acquire(self): now = time.time() # Add new tokens based on time passed self.tokens = min( self.tokens + (now - self.last_update) * self.tokens_per_second, self.tokens_per_second ) self.last_update = now if self.tokens >= 1: self.tokens -= 1 return True return False
Best Practices and Legal Considerations
When implementing Cloudflare bypass solutions, consider these best practices:
- Respect robots.txt: Always check and follow the website's crawling policies
- Implement backoff strategies: Use exponential backoff when encountering errors
- Monitor response codes: Track response patterns to adjust your approach
- Cache results: Implement appropriate caching to reduce request frequency
Legal Framework
Consider these legal aspects when implementing automated access solutions:
- Review and comply with the website's Terms of Service
- Implement data retention policies that align with GDPR and similar regulations
- Document your implementation's compliance measures
- Consider obtaining legal counsel for large-scale operations
Monitoring and Maintenance
Implement these monitoring practices to maintain reliable access:
- Track success rates across different proxy providers
- Monitor changes in Cloudflare's detection patterns
- Keep browser fingerprints and User-Agent strings updated
- Regularly rotate and validate proxy pools
Common Pitfalls to Avoid
- Using outdated User-Agent strings
- Failing to handle JavaScript challenges
- Ignoring geolocation consistency
- Not implementing proper error handling
- Overlooking TLS fingerprinting
From the Field: Developer Experiences
Technical discussions across various platforms reveal that developers encounter Cloudflare Error 1020 in different contexts, with varying levels of complexity in their bypass attempts. Engineers working on automated access solutions have documented several key findings about Cloudflare's detection mechanisms.
A recurring theme in developer forums is the distinction between browser-based and programmatic access. Many developers report that while standard browser requests work flawlessly, attempts using tools like Postman or Python's requests library trigger the 1020 error immediately. This behavior points to Cloudflare's sophisticated fingerprinting at the TLS layer, rather than just application-level detection. As one senior developer notes, the distinction lies in the TLS handshake patterns, which differ significantly between browsers and automated tools.
Implementation experiences vary based on the specific use case. Some developers report success with headless browser solutions, though with important caveats. When using tools like Selenium or Puppeteer in headless mode, many find that default configurations get blocked, while proper browser fingerprinting and careful configuration of User-Agent strings can help bypass detection. However, there's ongoing debate about the resource overhead of browser-based solutions versus lighter-weight approaches.
Performance considerations emerge as a significant factor in choosing bypass strategies. While headless browsers provide the most reliable bypass mechanism, developers working on large-scale operations express concerns about resource usage and scaling costs. Some teams report success with hybrid approaches, using lightweight requests for basic operations and falling back to browser-based solutions for more challenging scenarios.
The community generally acknowledges that Cloudflare's protection evolves continuously, making static solutions unreliable in the long term. Many developers advocate for flexible, multi-layered approaches that can adapt to changing detection patterns. There's also growing recognition that paying for specialized bypass services may be more cost-effective than maintaining custom solutions, especially for production-scale operations.
Conclusion
Successfully handling Cloudflare Error 1020 requires a multi-faceted approach combining technical solutions with responsible access practices. Whether you're a regular user or a developer implementing automated access, understanding the underlying mechanisms and available solutions is crucial for maintaining reliable access to Cloudflare-protected resources.
For more information about Cloudflare's security measures and updates, visit the official Cloudflare documentation.
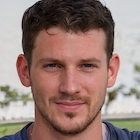