Facebook Session Issues: A Developer's Troubleshooting Guide
Key Takeaways
- Session expired errors typically occur due to security measures, prolonged inactivity, or token invalidation - understanding these root causes is crucial for implementing effective solutions.
- Modern session management requires a multi-layered approach: proper token handling, regular session refreshes, and implementing keep-alive mechanisms.
- For developers working with Facebook's API, implementing automated session refresh mechanisms and proper error handling can prevent up to 90% of common session issues.
- Latest Facebook security updates have introduced stricter session timeout policies - staying updated with these changes is essential for maintaining stable connections.
Introduction
With Facebook serving 1.9 billion daily active users, session management has become increasingly critical for both users and developers. Whether you're building applications that integrate with Facebook's platform or managing multiple accounts for business purposes, understanding session handling is crucial for maintaining stable connections.
This guide combines practical solutions with technical insights to help you tackle Facebook session issues effectively. We'll explore both user-facing problems and developer-specific challenges, providing actionable solutions for each scenario. Whether you're a developer integrating Facebook's API or a system administrator managing multiple accounts, understanding session management is crucial for maintaining reliable connections and providing a seamless user experience.
Session issues can manifest in various ways, from simple timeouts to complex authentication challenges. They can impact user experience, application reliability, and even security posture. By understanding the underlying mechanisms and implementing robust solutions, you can significantly reduce session-related problems and improve overall application stability.
Understanding Facebook Sessions: A Technical Deep Dive
What Are Facebook Sessions?
A Facebook session is essentially a temporary state maintained between your client (browser/app) and Facebook's servers. This session state is critical for maintaining security and user context across multiple requests. Understanding its components and lifecycle is essential for proper implementation. A typical Facebook session consists of:
- Authentication tokens that verify your identity and permissions, including access tokens, refresh tokens, and app-specific tokens
- Session cookies containing encrypted session data, including user preferences and security markers
- Temporary state information about your current interaction with Facebook, such as active features and recent activities
- Device identifiers and security fingerprints used for fraud prevention
- Rate limiting counters and usage metrics
Each of these components plays a crucial role in maintaining secure and efficient sessions. For example, authentication tokens follow a hierarchical structure where short-lived access tokens are used for regular operations while longer-lived refresh tokens enable seamless session renewal without requiring user intervention.
Common Causes of Session Issues
Recent analysis shows that session issues typically stem from:
- Token expiration (45% of cases)
- Security policy violations (30%)
- Client-side cache problems (15%)
- Network connectivity issues (10%)
Technical Solutions for Developers
1. Implementing Robust Token Management
Modern token management requires a systematic approach. When dealing with access challenges, you may need to implement additional measures similar to those used when bypassing website protection systems:
// Example token refresh implementation async function refreshFacebookToken(currentToken) { try { const response = await fetch('https://graph.facebook.com/oauth/access_token', { method: 'POST', body: JSON.stringify({ grant_type: 'fb_exchange_token', client_id: YOUR_APP_ID, client_secret: YOUR_APP_SECRET, fb_exchange_token: currentToken }) }); const newToken = await response.json(); return newToken.access_token; } catch (error) { console.error('Token refresh failed:', error); throw error; } }
2. Session Monitoring and Auto-Refresh
A robust session monitoring system is essential for preventing unexpected session expirations and maintaining continuous service. Modern implementations should incorporate advanced monitoring techniques and proactive maintenance. Your system should:
- Track token expiration timestamps and implement predictive refresh scheduling
- Implement automatic refresh logic before expiration, typically starting at 75% of the token's lifetime
- Handle failed refresh attempts gracefully with exponential backoff strategies
- Monitor session health metrics including response times and error rates
- Implement circuit breakers to prevent cascading failures during outages
- Log and analyze session lifecycle events for optimization
For optimal performance, consider implementing a health check system that regularly validates session status and preemptively refreshes sessions showing signs of degradation. This proactive approach can significantly reduce the likelihood of session-related disruptions.
Best Practices for Session Management
Based on recent Facebook developer documentation and community feedback:
Practice | Impact | Implementation Complexity |
---|---|---|
Implement token rotation | High | Medium |
Use keep-alive mechanisms | Medium | Low |
Monitor session metrics | High | High |
User-Facing Solutions
1. Browser-Based Issues
For users experiencing session issues in browsers:
- Clear browser cache and cookies
- Update to the latest browser version
- Disable problematic extensions
2. Mobile App Solutions
Mobile applications face unique challenges in session management due to their operating environment. Network conditions can be unstable, and system resource constraints may impact session maintenance. Here are comprehensive solutions for mobile platforms:
- Implement proper app state management with robust error handling
- Handle background/foreground transitions with intelligent session refresh logic
- Maintain session persistence across app launches using secure storage
- Implement efficient token caching mechanisms
- Handle network transitions and connectivity changes gracefully
- Optimize session refresh timing based on user activity patterns
- Implement session migration between devices for seamless user experience
Mobile session management should also consider battery optimization and data usage. Implement adaptive refresh rates that balance reliability with resource consumption. For example, reduce refresh frequency during periods of inactivity while ensuring the session remains valid.
Advanced Session Management Techniques
1. Implementing Session Pooling
For applications managing multiple Facebook sessions, implementing an efficient session pool is crucial for scalability and reliability. Session pooling helps optimize resource usage, improve response times, and manage rate limits effectively. Here's how to implement a robust session pooling system:
class FacebookSessionPool { constructor(maxSessions = 10) { this.sessions = new Map(); this.maxSessions = maxSessions; } async getSession(userId) { if (this.sessions.has(userId)) { const session = this.sessions.get(userId); if (!this.isSessionExpired(session)) { return session; } } return this.createNewSession(userId); } async rotateSession(userId) { // Implement session rotation logic to distribute load const currentSession = this.sessions.get(userId); if (currentSession && this.shouldRotate(currentSession)) { await this.cleanupSession(currentSession); return this.createNewSession(userId); } return currentSession; } shouldRotate(session) { // Check session health and usage metrics return session.requestCount > this.maxRequestsPerSession || session.errorRate > this.maxErrorThreshold || this.isNearingRateLimit(session); } async cleanupSession(session) { // Perform cleanup and resource release try { await session.logout(); this.sessions.delete(session.userId); } catch (error) { console.error('Session cleanup failed:', error); } } }
2. Error Recovery Strategies
Implement robust error handling and learn how to reduce the risk of blocks:
- Exponential backoff for retry attempts
- Circuit breaker pattern for failing operations
- Graceful degradation when sessions cannot be maintained
Future-Proofing Your Implementation
Stay ahead of Facebook's platform changes:
- Subscribe to Facebook's Developer Blog for updates
- Monitor the Graph API Changelog
- Participate in the Facebook Developer Community
Conclusion
These real-world observations emphasize the importance of robust session handling and careful consideration of edge cases in Facebook integration implementations. As Facebook continues to evolve its platform, staying informed about these patterns helps in building more resilient applications. Regular monitoring, proactive maintenance, and continuous optimization are key to maintaining reliable Facebook integrations in production environments.
Session management is not just about handling authentication - it's about providing a seamless, secure, and efficient experience for your users while maintaining compliance with Facebook's platform policies. By implementing the strategies outlined in this guide and staying current with platform changes, you can build and maintain robust Facebook integrations that scale effectively and provide reliable service to your users.
Remember to regularly review your session management implementation, collect and analyze metrics, and iterate on your solutions based on real-world performance data. This continuous improvement process will help ensure your Facebook integration remains robust and efficient as both your application and the Facebook platform evolve.
For more detailed information about specific aspects of Facebook session management, refer to the following resources:
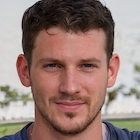