LinkedIn Data Extraction: A Developer's Guide to Ethical Scraping
Key Takeaways
- Modern LinkedIn data extraction requires a balanced approach between automation and responsible practices, with a focus on respecting rate limits and user privacy
- Successful implementation combines proper tools (Selenium, BeautifulSoup), proxy management, and ethical guidelines to ensure sustainable data collection
- Understanding LinkedIn's data structure and API limitations helps in building resilient extraction solutions that comply with platform policies
- Regular monitoring and adaptation of extraction strategies are crucial as LinkedIn frequently updates its platform security measures
- Using a combination of official APIs and ethical scraping methods provides the most reliable and sustainable approach to data collection
Understanding LinkedIn Data Extraction
LinkedIn remains the world's largest professional network, with over 950 million members as of early 2025. This vast repository of professional data presents immense opportunities for businesses, researchers, and developers. However, accessing this data requires a careful balance between technical capability and ethical responsibility. For reliable access to LinkedIn data, many developers leverage specialized LinkedIn proxies to ensure stable and compliant data collection.
This guide will walk you through modern approaches to LinkedIn data extraction, focusing on sustainable practices that respect both platform guidelines and user privacy. As part of a comprehensive data scraping infrastructure, these techniques can be invaluable for businesses and researchers.
The Evolution of LinkedIn Data Access
LinkedIn's approach to data access has evolved significantly over the years:
- 2015-2018: Relatively open access with basic rate limiting
- 2019-2021: Introduction of stricter anti-scraping measures
- 2022-2024: Implementation of AI-powered detection systems
- 2025: Advanced protection mechanisms with machine learning-based blocking
Understanding the Legal Framework
When implementing LinkedIn data extraction, it's crucial to understand the legal landscape. The landmark HiQ Labs v. LinkedIn case established important precedents regarding public data scraping. However, developers should still maintain strict compliance with platform policies and data protection regulations. Understanding common web scraping mistakes can help you avoid legal and technical pitfalls.
Technical Approaches to Data Extraction
1. Official LinkedIn APIs
The most reliable method is using LinkedIn's official APIs:
- Marketing Developer Platform: For advertising and marketing automation
- Talent Solutions: For recruitment and hiring
- Partner Programs: For authorized data partners
Learn more about official APIs at LinkedIn's Developer Portal.
2. Ethical Scraping Techniques
When official APIs don't meet your needs, here's a responsible approach to data collection. Understanding how to avoid blocking while scraping is essential for sustainable data extraction:
import asyncio from playwright.async_api import async_playwright from bs4 import BeautifulSoup async def extract_profile_data(url): async with async_playwright() as p: browser = await p.chromium.launch(headless=True) context = await browser.new_context() # Implement reasonable delays await asyncio.sleep(2) page = await context.new_page() await page.goto(url) # Extract public data only content = await page.content() soup = BeautifulSoup(content, 'html.parser') # Clean up await browser.close() return parsed_data
3. Rate Limiting and Request Management
Implement proper rate limiting to avoid platform restrictions. Understanding common proxy error codes and solutions will help you handle issues effectively:
class LinkedInRateLimiter: def __init__(self): self.requests = [] self.max_requests = 100 # per hour self.window = 3600 # seconds async def can_make_request(self): current_time = time.time() self.requests = [req for req in self.requests if current_time - req < self.window] return len(self.requests) < self.max_requests
Best Practices for Sustainable Data Collection
1. Technical Implementation
- Implement proper proxy rotation and management
- Use exponential backoff for failed requests
- Maintain session management for authentication
- Monitor response patterns to avoid detection
2. Ethical Considerations
- Respect user privacy and data protection regulations
- Only collect publicly available information
- Implement data retention and deletion policies
- Maintain transparency about data usage
Advanced Data Extraction Strategies
1. Profile Data Extraction
When extracting profile data, focus on publicly available information such as:
- Professional experience
- Educational background
- Skills and endorsements
- Public activity and posts
2. Company Data Collection
For company data, prioritize:
- Company overview and details
- Employee count and growth trends
- Job postings and requirements
- Industry insights and updates
Error Handling and Recovery
Implement robust error handling mechanisms to manage common scenarios:
async def handle_linkedin_errors(response): if response.status == 429: # Too Many Requests await implement_backoff() elif response.status == 403: # Forbidden await rotate_proxy() elif response.status == 500: # Server Error await retry_with_delay()
Future Considerations
As LinkedIn continues to evolve, stay prepared for:
- Enhanced AI-based detection systems
- Stricter rate limiting and access controls
- New API endpoints and capabilities
- Updated terms of service and usage policies
Performance Optimization
Optimize your data extraction pipeline through:
- Efficient request batching
- Smart caching strategies
- Parallel processing where appropriate
- Resource utilization monitoring
Field Notes: Developer Experiences
Technical discussions across various platforms reveal a complex landscape of LinkedIn data extraction practices, with developers sharing both successes and challenges in their implementations.
Engineering teams consistently highlight the importance of approach selection based on use case. While some developers report success with basic scraping tools like Selenium and BeautifulSoup for public data, others emphasize the need for more sophisticated solutions when dealing with larger-scale operations. A notable trend among experienced practitioners is the recommendation to use browser extensions for personal tools, as they leverage existing user sessions and reduce detection risks.
Security considerations dominate many technical discussions. Developers with hands-on experience frequently mention LinkedIn's sophisticated detection systems, particularly for logged-in scraping attempts. Several teams report success with proxy rotation and browser fingerprint management, though they caution that implementation complexity increases significantly at scale. Interestingly, developers working with public data report fewer issues when respecting rate limits and focusing on publicly accessible endpoints.
Legal and ethical considerations feature prominently in community discussions. While some developers reference the 2022 HiQ Labs vs LinkedIn case that established the legality of scraping public data, others emphasize the importance of respecting LinkedIn's terms of service, particularly when dealing with private data or logged-in sessions. Many experienced practitioners advocate for a hybrid approach that combines official APIs with ethical scraping practices.
The community generally agrees that successful LinkedIn data extraction requires a balance between technical capability and responsible practices. Most experienced developers recommend starting with official APIs where possible, only moving to scraping solutions when necessary, and always implementing robust rate limiting and error handling regardless of the chosen approach.
Conclusion
Successful LinkedIn data extraction requires a balanced approach that combines technical expertise with ethical considerations. By following the guidelines and best practices outlined in this guide, you can build sustainable data collection systems that respect both platform policies and user privacy.
Remember to stay updated with LinkedIn's terms of service and regularly adjust your approaches as the platform evolves. The future of data extraction lies in responsible practices that create value while maintaining trust.
Additional Resources
To further expand your knowledge of LinkedIn data extraction and web scraping, here are some valuable external resources:
- Requests Library Documentation - Essential for making HTTP requests in Python
- Playwright for Python - Modern web automation library
- Beautiful Soup Documentation - Complete guide to HTML parsing
- AIOHTTP Documentation - Async HTTP client/server framework
- Selenium Documentation - Comprehensive browser automation guide
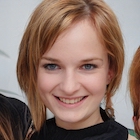