JavaScript Rendering in Web Scraping: Beyond Static Content
Key Takeaways
- Traditional scraping methods fail with JavaScript-rendered content, requiring specialized tools like headless browsers
- Puppeteer, Playwright, and Selenium are the leading JavaScript rendering solutions with different performance profiles
- Post-rendering data extraction typically uses DOM selectors via tools like Cheerio or built-in browser methods
- Rendering delays, proxy usage, and browser fingerprint management are crucial for successful JS scraping
Introduction: The JavaScript Rendering Challenge
Modern websites increasingly rely on JavaScript to deliver dynamic, interactive content. According to a study by HTTP Archive, over 96% of websites use JavaScript to some extent, with the average site loading approximately 30 scripts. For web scrapers, this poses a significant challenge – traditional HTTP requests only retrieve the initial HTML, missing all the dynamically loaded content.
Consider this example from Mint Mobile's website:
With JavaScript disabled: Only bare-bones HTML structure, missing pricing, product details, and interactive elements.
With JavaScript enabled: Complete product information, pricing details, interactive buttons, and dynamically loaded images.
This dramatic difference highlights why JavaScript rendering has become essential for effective web scraping. In this comprehensive guide, we'll explore how to overcome these challenges using various tools and strategies.
Understanding JavaScript-Rendered Web Pages
The Difference Between Static and Dynamic Content
Before diving into solutions, it's important to understand what makes JavaScript-rendered content different:
- Static Web Pages: All content is fully present in the initial HTML document delivered by the server
- JavaScript-Rendered Pages: Essential content is loaded or generated after the initial page load through:
- AJAX calls that fetch data from APIs
- DOM manipulation that creates new elements
- React, Vue, or Angular frameworks that build the interface dynamically
- Event-triggered content that appears based on scrolling, clicking, or other interactions
According to a report by W3Techs, 97.4% of websites use client-side JavaScript, and Single Page Applications (SPAs) built with frameworks like React, Vue, and Angular now represent approximately 65% of modern web applications.
Impact on Scraping Strategy
The widespread adoption of JavaScript rendering requires a significant shift in scraping approaches:
Traditional Scraping | JavaScript-Aware Scraping |
---|---|
Simple HTTP requests | Requires browser environment |
Fast (milliseconds per request) | Slower (seconds per request) |
Low resource usage | Higher memory and CPU requirements |
Straightforward scaling | More complex scaling considerations |
JavaScript Rendering Tools for Web Scraping
Several tools have emerged to address the JavaScript rendering challenge. Each offers different capabilities, performance characteristics, and ease of use.
Headless Browser Solutions
Puppeteer is a Node.js library that provides a high-level API to control Chrome or Chromium programmatically. It's maintained by the Chrome DevTools team and offers comprehensive browser automation capabilities.
const puppeteer = require('puppeteer'); (async () => { // Launch a headless browser const browser = await puppeteer.launch(); const page = await browser.newPage(); // Navigate to the target URL await page.goto('https://example.com'); // Wait for specific content to load await page.waitForSelector('.dynamic-content'); // Extract data from the fully rendered page const data = await page.evaluate(() => { return { title: document.querySelector('h1').innerText, items: Array.from(document.querySelectorAll('.item')).map(el => el.innerText) }; }); console.log(data); await browser.close(); })();
Strengths: Excellent Chrome/Chromium integration, comprehensive API, strong community support
Limitations: Limited to Chrome/Chromium, higher resource requirements than traditional HTTP requests
Playwright
Developed by Microsoft, Playwright extends the capabilities of Puppeteer to support multiple browsers (Chromium, Firefox, and WebKit). It offers improved reliability and cross-browser testing.
const { chromium } = require('playwright'); (async () => { // Launch a headless browser const browser = await chromium.launch(); const page = await browser.newPage(); // Navigate to the target URL with network idle waiting await page.goto('https://example.com', { waitUntil: 'networkidle' }); // Extract data using Playwright's selector engine const title = await page.textContent('h1'); const items = await page.$$eval('.item', elements => elements.map(el => el.innerText) ); console.log({ title, items }); await browser.close(); })();
Strengths: Cross-browser support, modern API design, better automation capabilities than Puppeteer
Limitations: Still relatively resource-intensive, requires careful management for large-scale scraping
Selenium
As the oldest browser automation tool in this list, Selenium offers the widest range of language bindings and browser support. It's more verbose than newer alternatives but remains a popular choice, especially for teams with existing Selenium expertise.
const { Builder, By, until } = require('selenium-webdriver'); (async function example() { let driver = await new Builder().forBrowser('chrome').build(); try { // Navigate to the target URL await driver.get('https://example.com'); // Wait for specific content to load await driver.wait(until.elementLocated(By.css('.dynamic-content')), 10000); // Extract data const title = await driver.findElement(By.css('h1')).getText(); const items = await Promise.all( (await driver.findElements(By.css('.item'))).map(el => el.getText()) ); console.log({ title, items }); } finally { await driver.quit(); } })();
Strengths: Mature ecosystem, wide language support, excellent for complex browser interactions
Limitations: More verbose API, slower than Puppeteer/Playwright, higher maintenance requirements
Framework-Specific Tools
For Python developers using the Scrapy framework, several middleware options exist to add JavaScript rendering capabilities:
- Scrapy-Splash: Integrates the Splash rendering service with Scrapy
- Scrapy-Playwright: Combines Scrapy with Playwright for multi-browser support
- Scrapy-Selenium: Adds Selenium support to Scrapy pipelines
These extensions allow developers to maintain their existing Scrapy codebase while adding JavaScript rendering capabilities.
Advanced JavaScript Rendering Techniques
Optimizing Rendering Performance
JavaScript rendering is inherently more resource-intensive than traditional scraping. Here are strategies to optimize performance:
Controlled Rendering
Not all JavaScript-heavy sites require full rendering. You can optimize by:
- Selectively executing scripts: Disable non-essential scripts to speed up rendering
- Using render delay: Set appropriate wait times based on page complexity
- Waiting for specific elements: Rather than arbitrary delays, wait for critical selectors
// Example with Puppeteer - waiting for specific content await page.goto('https://example.com', { waitUntil: 'domcontentloaded' }); await page.waitForSelector('#dynamic-content', { visible: true }); // Example with ZenRows - using specific instructions const params = { url: 'https://example.com', js_render: 'true', js_instructions: '[{"wait_for": "#dynamic-content"}]' };
Resource Blocking
In a performance study by Ahrefs, blocking non-essential resources reduced rendering time by up to 60% while still retrieving necessary data.
// Example with Puppeteer - blocking images, fonts, and stylesheets await page.setRequestInterception(true); page.on('request', (req) => { if ( req.resourceType() === 'image' || req.resourceType() === 'font' || req.resourceType() === 'stylesheet' ) { req.abort(); } else { req.continue(); } });
Executing Custom JavaScript
One of the most powerful capabilities of JavaScript rendering tools is the ability to execute custom JavaScript in the context of the page. This enables:
- Extracting data from complex structures
- Interacting with page elements
- Bypassing client-side protections
Here's an example using Puppeteer to scroll through an infinite-loading page and extract all items:
// Scroll to bottom and collect all items from infinite-loading page const items = await page.evaluate(async () => { const results = []; // Helper function to scroll to bottom const scrollToBottom = () => { window.scrollTo(0, document.body.scrollHeight); return new Promise(resolve => setTimeout(resolve, 1000)); }; // Get current height let lastHeight = document.body.scrollHeight; // Scroll and check for new content while (true) { await scrollToBottom(); // Collect items currently visible document.querySelectorAll('.item').forEach(item => { results.push({ title: item.querySelector('.title').innerText, price: item.querySelector('.price').innerText }); }); // Check if we've reached the end if (document.body.scrollHeight === lastHeight) { break; } lastHeight = document.body.scrollHeight; } return results; });
Handling Anti-Bot Measures
Modern websites employ various anti-bot techniques that can interfere with JavaScript rendering. According to Imperva's Bad Bot Report, 30.2% of all web traffic comes from bots, prompting increasing sophistication in detection methods.
Common detection methods include:
- Browser fingerprinting
- Mouse movement and interaction tracking
- Behavioral analysis
- IP reputation checking
- CAPTCHA and other interactive challenges
To counter these measures:
Use Premium Proxies
Residential proxies that route traffic through real consumer devices can significantly improve success rates compared to datacenter IPs.
Implement Browser Fingerprint Management
// Example with Puppeteer - modifying browser fingerprint await page.evaluateOnNewDocument(() => { // Override the navigator properties Object.defineProperty(navigator, 'webdriver', { get: () => false }); // Add language plugins to appear more like a regular browser Object.defineProperty(navigator, 'languages', { get: () => ['en-US', 'en'] }); // Add a fake user agent if needed Object.defineProperty(navigator, 'userAgent', { get: () => 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36' }); });
Consider API Solutions
Services like ZenRows maintain sophisticated anti-detection mechanisms that are constantly updated, often achieving higher success rates than self-managed solutions.
Real-World Use Cases and Best Practices
Case Study: E-commerce Product Monitoring
A retail analytics company needed to track pricing and inventory across 50+ competitor websites, many using React and Angular frameworks. Their initial approach with traditional scraping failed to capture dynamic pricing changes.
Solution: They implemented a Playwright-based scraping infrastructure with the following optimizations:
- Browser recycling to reduce startup overhead
- Selective rendering based on site complexity
- Custom extraction scripts tailored to each target site
- Distributed cloud infrastructure across multiple regions
Results: 94% success rate (up from 37% with traditional methods), with 60% reduction in per-page processing time after optimization.
Best Practices for JavaScript Rendering at Scale
1. Implement Intelligent Caching
Not all pages need to be rendered with every request. Implement a caching strategy based on:
- Content update frequency
- Resource constraints
- Acceptable data freshness
2. Prioritize Error Handling and Retry Logic
JavaScript rendering is inherently more prone to failures than traditional HTTP requests. Implement robust error handling with:
- Intelligent retry mechanisms with exponential backoff
- Alternate rendering strategies as fallbacks
- Comprehensive logging for debugging
3. Monitor and Adapt
Websites constantly evolve their technologies and anti-bot measures. Implement:
- Regular success rate monitoring
- Automated tests against key targets
- Alerting for sudden changes in success patterns
Field Notes: JavaScript Rendering in Web Scraping
The ongoing conversation in tech communities suggests JavaScript rendering remains both a challenge and an opportunity in web scraping. Experienced developers frequently recommend browser automation tools like Selenium as a first-line solution when encountering dynamic content, while JavaScript developers tend to gravitate toward Puppeteer or Playwright based on their ecosystem familiarity. These preferences highlight the importance of leveraging existing expertise when selecting a rendering approach.
A competing perspective that has gained significant traction focuses on efficiency through analyzing network requests rather than rendering entire pages. Many engineers advocate investigating XHR requests in browser developer tools to identify underlying JSON data sources that power dynamic content. This approach bypasses the resource-intensive process of running a full browser instance, potentially offering significant performance improvements, though it requires more initial investigation and a deeper understanding of how the target site functions.
Real-world implementations have exposed technical hurdles not immediately apparent from documentation, including environment-specific issues like event loop conflicts and URL construction problems with timestamp handling. Meanwhile, the community remains divided on the trade-off between convenience and control, with commercial services offering simplicity for time-sensitive projects while custom implementations provide the fine-tuning capabilities needed for complex scraping operations.
What becomes clear across these varied perspectives is that JavaScript rendering in web scraping requires a contextual approach rather than a universal solution. The ideal method depends on factors like the target site's architecture, the volume of data needed, available resources, and developer expertise. Successful implementations typically come from understanding both the strengths and limitations of each approach and selecting the one that best aligns with project-specific requirements.
The Future of JavaScript Rendering in Web Scraping
The web continues to evolve toward more JavaScript-intensive applications. Several trends will shape the future of JavaScript rendering for web scraping:
WebAssembly and New Browser Technologies
As WebAssembly (WASM) adoption increases, web applications will leverage this technology for performance-critical code. Scraping tools will need to adapt to interpret and execute WASM modules.
AI-Powered Rendering Optimization
Machine learning algorithms are being developed to predict optimal rendering strategies based on target site characteristics, potentially reducing resource requirements while maintaining high success rates.
Enhanced Anti-Bot Technologies
Expect increasingly sophisticated browser fingerprinting and behavioral analysis to detect automated browsers. The arms race between scrapers and anti-bot technologies will continue to intensify.
Conclusion: Choosing the Right JavaScript Rendering Strategy
JavaScript rendering has become an essential component of modern web scraping. The right approach depends on your specific requirements:
- For small-scale projects: Self-hosted solutions like Puppeteer or Playwright offer flexibility and control
- For large-scale operations: API-based solutions provide better scalability and maintenance
- For maximum flexibility: Hybrid approaches combining multiple techniques can optimize for both performance and success rate
Regardless of the chosen strategy, successful JavaScript rendering in web scraping requires a deep understanding of how modern websites function, combined with thoughtful implementation and continuous refinement.
By leveraging the techniques described in this guide, you can overcome the challenges of JavaScript-rendered content and successfully extract valuable data from even the most complex modern websites. To avoid getting blocked while scraping, be sure to check out our guide on how to scrape a website without getting blocked, and learn from common web scraping mistakes beginners make to ensure your scraping operations are effective and efficient.
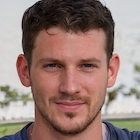