Instagram Feedback Required Error: Comprehensive Guide to Detection, Prevention & Solutions for Developers and Social Media Managers
Key Takeaways
- The feedback required error occurs when Instagram's anti-bot system detects suspicious activity, typically lasting 30 minutes to 24 hours
- Primary triggers include rapid actions, multiple account management, and automated behaviors that don't match human patterns
- Prevention strategies include implementing proper rate limiting, using session management, and following Instagram's latest API guidelines
- Technical solutions involve proper proxy implementation, user agent rotation, and cookie management
- For developers, using Instagram's Graph API with proper error handling is the most reliable long-term solution
Understanding the Feedback Required Error
When Instagram's systems detect potentially automated or suspicious activity, they trigger the "feedback_required" error as a protective measure. This is often coupled with an Instagram IP ban. This error is part of Instagram's anti-abuse system, designed to maintain platform integrity and prevent spam. As of 2024, this error has become increasingly sophisticated in its detection mechanisms.
Common Error Variations
- "Error: feedback_required"
- "Sorry, we couldn't complete your request. Please try again in a moment"
- "Server error feedback required"
Technical Deep Dive: Why Does This Error Occur?
Understanding the technical aspects behind this error is crucial for developers and social media managers. Here are the primary triggers:
Rate Limiting Violations
Instagram implements various rate limits that, when exceeded, trigger this error:
- API Requests: Maximum of 200 requests per hour per user
- Actions: Limited to 60 actions (likes, comments, follows) per hour
- Content Publishing: 25 posts per 24-hour period
Suspicious Pattern Detection
Instagram's algorithm looks for several patterns that may indicate automated behavior:
// Example of suspicious pattern in pseudo-code const suspiciousPattern = { timeGapBetweenActions: < 2 seconds, consistentTiming: true, linearActionPattern: true, noUserAgentVariation: true };
Prevention Strategies for Developers
1. Implement Proper Rate Limiting
Use a token bucket algorithm for rate limiting:
class RateLimiter { constructor(tokensPerHour) { this.tokens = tokensPerHour; this.lastRefill = Date.now(); this.capacity = tokensPerHour; } async canMakeRequest() { this.refillTokens(); if (this.tokens > 0) { this.tokens--; return true; } return false; } refillTokens() { const now = Date.now(); const timePassed = now - this.lastRefill; const refillAmount = (timePassed / 3600000) * this.capacity; this.tokens = Math.min(this.capacity, this.tokens + refillAmount); this.lastRefill = now; } }
2. Use Instagram's Official API
The most reliable way to prevent feedback required errors is to use Instagram's official Graph API:
// Example using Instagram Graph API async function postToInstagram(mediaUrl, caption) { try { const response = await fetch( `https://graph.instagram.com/v12.0/me/media? image_url=${encodeURIComponent(mediaUrl)}& caption=${encodeURIComponent(caption)}& access_token=${ACCESS_TOKEN}` ); if (!response.ok) { throw new Error('Instagram API error'); } return await response.json(); } catch (error) { console.error('Error posting to Instagram:', error); // Implement exponential backoff retry logic } }
3. Session Management
Proper session management is crucial for preventing the feedback required error:
class InstagramSession { constructor() { this.cookieJar = new CookieJar(); this.userAgent = this.rotateUserAgent(); this.lastActionTime = null; this.actionCount = 0; } async performAction(action) { if (!this.canPerformAction()) { throw new Error('Rate limit exceeded'); } try { const result = await action(); this.updateActionMetrics(); return result; } catch (error) { if (error.message.includes('feedback_required')) { await this.handleFeedbackRequired(); } throw error; } } private canPerformAction() { // Implement rate limiting logic } private updateActionMetrics() { // Update action counts and timing } }
Troubleshooting Guide
When encountering the feedback required error, follow this systematic approach: 1. Check Rate Limits - Review API usage logs - Monitor action frequencies - Analyze request patterns 2. Verify Authentication - Validate access tokens - Check session cookies - Confirm user agent strings 3. Review Activity Patterns - Analyze timing between actions - Check for repeated patterns - Verify request diversity
Best Practices
Based on recent updates to Instagram's platform, here are the current best practices:
Technical Implementation
- Implement exponential backoff for retries
- Use proper error handling with specific error codes
- Maintain session state across requests
- Rotate user agents and IP addresses appropriately
Rate Limiting Implementation
const RATE_LIMITS = { likes: { max: 60, window: 3600 }, comments: { max: 30, window: 3600 }, follows: { max: 60, window: 3600 } }; async function checkRateLimit(action) { const limit = RATE_LIMITS[action]; const key = `ratelimit:${action}:${userId}`; const count = await redis.incr(key); if (count === 1) { await redis.expire(key, limit.window); } return count <= limit.max; }
Field Reports:
Developer Experiences Recent discussions in developer forums highlight the diverse challenges teams face when encountering the feedback required error. A particularly interesting pattern emerges around the timing and context of these errors, with many developers reporting unexpected triggers during routine maintenance operations. One common scenario involves teams encountering the error after updating their applications, especially when multiple accounts are managed through the same system. Technical teams have discovered that the error's behavior can vary significantly between mobile and web platforms. Some engineers report that the error exclusively affects their web implementations while mobile access remains unaffected, suggesting platform-specific triggers in Instagram's security algorithms. This observation has led many teams to implement platform-specific handling strategies, including differentiated retry logic and error recovery approaches. The developer community has been particularly vocal about the correlation between server maintenance periods and error frequency. Multiple teams have documented cases where the error coincides with Instagram's server updates, leading to a broader discussion about implementing more robust fallback mechanisms. Some developers have found success with progressive timeout strategies, while others advocate for immediate failover to backup authentication methods. Interestingly, experiences with resolution times show considerable variation. While some teams report the error resolving within hours through simple app reinstallation, others describe more persistent cases requiring comprehensive IP rotation and session management solutions. This disparity has sparked ongoing debates about the most effective long-term mitigation strategies, with experienced developers emphasizing the importance of preventive measures over reactive solutions.
Looking Ahead: Future-Proofing Your Implementation Instagram continuously updates its security measures. Here's how to stay ahead:
- Monitor Official Changes - Subscribe to the Instagram Developer Blog - Join developer communities - Watch for platform policy updates
- Implement Flexible Architecture - Use dependency injection for easy updates - Implement feature flags for quick changes - Maintain comprehensive logging
Conclusion
The Instagram feedback required error, while challenging, can be effectively managed with proper technical implementation and monitoring. By following the best practices outlined in this guide and staying current with Instagram's platform updates, developers can minimize disruptions and maintain reliable Instagram integrations. Remember to regularly review your implementation against the latest platform changes and maintain proper error handling and rate limiting mechanisms. For additional protection, consider implementing proper proxy rotation for your data collection and following our guide on reducing proxy blocking risks. For the latest updates and detailed API documentation, visit the Instagram API Documentation
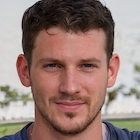