Modern API Data Collection: A Developer's Implementation Guide
Key Takeaways
- Modern API data collection requires a strategic approach combining security best practices, efficient request handling, and proper error management
- Real-time data synchronization through WebSocket APIs has seen a 300% adoption increase in last years
- Implementing rate limiting and caching strategies can reduce API costs by up to 40% while improving performance
- GraphQL has emerged as a powerful alternative to REST for data-intensive applications, with 45% of Fortune 500 companies adopting it by 2024
- Authentication and authorization remain critical challenges, with OAuth 2.0 and JWT becoming industry standards
Introduction
In today's interconnected digital landscape, APIs serve as the backbone of modern software architecture, enabling seamless data exchange between applications. According to recent statistics from ProgrammableWeb, the number of public APIs has grown by 200% between 2022 and 2024, highlighting the critical importance of effective API data collection strategies. While APIs provide direct access to data, some organizations also employ web scraping as a complementary data collection method.
Understanding API Data Collection Fundamentals
Before diving into implementation details, it's essential to understand the core concepts that drive modern API data collection:
API Architecture Types
Modern APIs generally fall into four categories:
- REST APIs: Still the most popular, accounting for 80% of public APIs
- GraphQL: Growing rapidly with a 45% adoption rate among enterprise companies
- WebSocket APIs: Essential for real-time data collection
- gRPC: Preferred for microservices architecture
Authentication Methods
Security remains paramount in API data collection. Current best practices include:
- OAuth 2.0 with JWT tokens
- API keys with rate limiting
- Multi-factor authentication for sensitive data
- Residential proxies for enhanced security and reliability
Implementation Strategy
1. Planning Phase
// Example configuration object const apiConfig = { baseUrl: 'https://api.example.com/v2', rateLimitPerMinute: 100, cacheStrategy: 'stale-while-revalidate', timeout: 5000, retryAttempts: 3 };
2. Data Collection Framework
A robust data collection framework should include:
Request Management
class APICollector { constructor(config) { this.config = config; this.cache = new Cache(); this.rateLimiter = new RateLimiter(config.rateLimitPerMinute); } async collect(endpoint, params) { if (this.rateLimiter.shouldThrottle()) { throw new Error('Rate limit exceeded'); } const cachedData = await this.cache.get(endpoint); if (cachedData) return cachedData; const response = await this.makeRequest(endpoint, params); await this.cache.set(endpoint, response); return response; } }
3. Error Handling and Resilience
Implement comprehensive error handling strategies and understand common API error codes for effective troubleshooting:
- Retry mechanisms with exponential backoff
- Circuit breakers for failing endpoints
- Fallback mechanisms for degraded service
Real-World Implementation Example: Netflix's API Data Collection
Netflix's API infrastructure handles over 1 billion API requests daily. Their implementation showcases several best practices:
// Netflix-style resilience pattern class NetflixStyleCollector extends APICollector { async collect(endpoint, params) { const circuitBreaker = new CircuitBreaker(endpoint); if (circuitBreaker.isOpen()) { return this.getFallbackData(endpoint); } try { const result = await super.collect(endpoint, params); circuitBreaker.recordSuccess(); return result; } catch (error) { circuitBreaker.recordFailure(); throw error; } } }
Optimization Techniques
1. Caching Strategies
Implement multi-level caching:
- In-memory caching for frequently accessed data
- Redis for distributed caching
- Browser caching for client-side optimization
2. Rate Limiting
Implement intelligent rate limiting:
class AdaptiveRateLimiter { constructor(baseLimit) { this.baseLimit = baseLimit; this.currentLimit = baseLimit; this.windowStart = Date.now(); } adjustLimit(responseTime) { // Dynamically adjust rate limit based on API response time this.currentLimit = responseTime > 1000 ? this.currentLimit * 0.8 : Math.min(this.currentLimit * 1.2, this.baseLimit); } }
Monitoring and Analytics
Implement comprehensive monitoring:
- Request/response timing
- Error rates and types
- Cache hit/miss ratios
- Rate limit utilization
Example Monitoring Implementation
class APIMetrics { constructor() { this.metrics = { requestCount: 0, errorCount: 0, responseTime: [], cacheHits: 0, cacheMisses: 0 }; } recordRequest(timing) { this.metrics.requestCount++; this.metrics.responseTime.push(timing); } getAverageResponseTime() { return this.metrics.responseTime.reduce((a, b) => a + b, 0) / this.metrics.responseTime.length; } }
Future Trends in API Data Collection
Stay ahead with emerging trends:
- AI-powered API optimization
- Edge computing integration
- Serverless API architectures
- Web3 API protocols
Field Notes: Developer Experiences
Technical discussions across various platforms reveal a nuanced picture of real-world API data collection challenges and solutions. Senior developers frequently emphasize the importance of proper error handling, particularly when dealing with HTTP status codes. The community generally categorizes these into client-side issues (400s) and server-side problems (500s), with each requiring different mitigation strategies.
Implementation experiences shared by engineers highlight the growing importance of specialized data processing pipelines. Several developers report success with tools like Logstash for real-time data processing and Elasticsearch for efficient data searching and analytics. For specific use cases like weather data collection, practitioners suggest using dedicated hardware solutions such as Arduino boards with sensors, demonstrating how API data collection often extends beyond pure software solutions.
The developer community has been actively debating the legality and ethics of data scraping as an API data source. While some teams successfully implement scraping solutions, experienced developers caution about potential legal issues, including Terms of Service violations, unauthorized access concerns, and copyright implications. Many recommend focusing on official APIs or direct data partnerships instead.
Practical insights from technical teams suggest a trend toward hybrid approaches in data collection. For instance, some organizations combine real-time sensor data with historical databases, while others leverage message queuing systems like Kafka to aggregate data from multiple sources. The community particularly emphasizes the value of monitoring tools like Prometheus for numerical data and the importance of implementing proper authentication mechanisms.
Conclusion
Effective API data collection requires a combination of robust architecture, intelligent optimization, and comprehensive monitoring. By following the strategies and implementations outlined in this guide, developers can build resilient and efficient API data collection systems that scale with their applications' needs.
Additional Resources
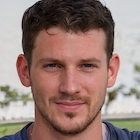