Rotating Proxies: The Ultimate Guide to Web Scraping at Scale in 2025
Key Takeaways
- Rotating proxies automatically cycle through IP addresses, helping web scrapers avoid detection and blocking while distributing requests across thousands of IPs.
- Smart rotation strategies (subnet diversity, performance tracking, weighted randomization) can significantly increase scraping success rates and resource efficiency.
- Residential proxies, though more expensive than datacenter proxies, offer substantially higher success rates because they appear as legitimate user devices.
- Modern anti-bot systems have evolved to detect patterns beyond IP addresses, requiring sophisticated rotation strategies that include browser fingerprinting and request timing.
- Using a proxy management service eliminates infrastructure complexity while providing access to larger IP pools and built-in anti-ban technologies.
What Are Rotating Proxies and Why Should You Care?
Web scraping at scale is increasingly challenging as websites implement sophisticated anti-bot systems to protect their data. One of the most effective techniques to overcome these obstacles is using rotating proxies, which has become practically essential for any serious web scraping operation in this year.
A rotating proxy is a server that automatically cycles your requests through a pool of different IP addresses, assigning a new IP for each connection or at regular intervals. This approach simulates multiple users accessing a website rather than numerous requests from a single source, helping you bypass rate limits and avoid IP bans.
The Evolution of Rotating Proxies
The concept of rotating proxies has evolved significantly since its inception. In the early days of web scraping, simple IP rotation was sufficient to avoid detection. However, as anti-bot technologies have become more sophisticated, rotating proxy solutions have had to adapt accordingly.
According to Imperva's report, sophisticated bad bots now account for 29.3% of all website traffic, a 6.1% increase from 2023. This has led to an arms race between web scrapers and anti-bot systems, with rotating proxies becoming increasingly complex and intelligent to avoid detection.
How Rotating Proxies Work
At a fundamental level, a rotating proxy works by intercepting your web requests and routing them through different IP addresses. Here's the process:
- You send a request to the rotating proxy server
- The proxy server selects an IP address from its pool
- Your request is forwarded to the target website through that IP
- The website responds to the proxy server
- The proxy server forwards the response back to you
- For subsequent requests, the proxy server selects a different IP address
This rotation happens automatically without requiring you to manually switch between proxies, which dramatically simplifies your scraping infrastructure.
Datacenter vs. Residential Rotating Proxies
When implementing rotating proxies, you have two main options: datacenter proxies and residential proxies. Understanding the differences is crucial for selecting the right solution for your needs.
Datacenter Proxies | Residential Proxies |
---|---|
Created in cloud servers/datacenters | Assigned to real residential devices via ISPs |
Not associated with ISPs or real users | Associated with legitimate residential connections |
Easily identifiable as proxies by sophisticated anti-bot systems | Appear as genuine user connections |
Lower cost ($0.5-$2 per IP/month) | Higher cost ($10-$20 per GB of traffic) |
Higher speeds and reliability | Variable speeds but better success rates |
Residential proxies achieve a 78% higher success rate on heavily protected websites compared to datacenter proxies, despite costing 5-10 times more. This price-performance tradeoff is a crucial consideration when planning your scraping strategy.
Why Rotating Proxies Are Essential for Web Scraping
Understanding why rotating proxies are vital for web scraping will help you appreciate their value and implement them effectively.
Distributed Request Load
The most obvious benefit of rotating proxies is distributing your requests across thousands (or millions) of IP addresses. Most websites implement some form of rate limiting that restricts the number of requests from a single IP address within a specific timeframe.
For example, LinkedIn restricts users to approximately 100 page views per hour from a single IP, while Amazon typically allows 1-2 requests per second before displaying CAPTCHAs. By using rotating proxies, you can easily scale your scraping operation to thousands of pages without hitting these limits.
Bypassing Advanced Anti-Bot Systems
Modern websites employ sophisticated anti-bot technologies that go beyond simple IP-based rate limiting. These systems analyze various signals to determine whether a request comes from a legitimate user or a bot:
- IP reputation and history
- Browser fingerprints and headers
- Request patterns and timing
- JavaScript execution capabilities
- Mouse movements and behavior patterns
Rotating proxies, especially when combined with browser fingerprinting rotation and proper request timing, can help bypass these systems by making your requests appear to come from different legitimate users.
Geo-Targeting and Localized Content Access
Many websites serve different content based on geographic location. Using rotating proxies with IPs from specific countries allows you to access location-specific content simultaneously.
This capability is particularly valuable for:
- Price comparison across different regions
- Verifying localized SEO rankings
- Accessing region-restricted content
- Testing international versions of websites
In a Journal of Big Data study, researchers found that pricing for identical products varied by up to 43% across different geographic locations, highlighting the importance of geo-diverse scraping capabilities.
Advanced Proxy Rotation Strategies
Simply rotating through proxies randomly isn't enough for modern web scraping. Sophisticated websites can detect basic rotation patterns. Here are advanced strategies to maximize your success rate:
Subnet-Aware Rotation
IP addresses are organized into subnets, which are groups of IPs sharing the same network prefix. Many anti-bot systems track requests at the subnet level, not just individual IPs.
For effective rotation, ensure consecutive requests use IPs from different subnets:
# Python example of subnet-aware rotation import random proxies = [ "xx.xx.123.1", "xx.xx.123.2", "xx.xx.124.1", "xx.xx.125.1", ] last_subnet = None def get_proxy(): global last_subnet attempts = 0 while attempts < 10: ip = random.choice(proxies) ip_subnet = ip.split('.')[2] if ip_subnet != last_subnet: last_subnet = ip_subnet return ip attempts += 1 # If we can't find a different subnet after 10 attempts, just return any proxy return random.choice(proxies)
Performance-Based Weighted Rotation
Not all proxies perform equally. Some may be slower, less reliable, or already flagged by target websites. A smart rotation strategy tracks proxy performance and adjusts selection probability accordingly.
Here's a simplified implementation of weighted rotation based on proxy performance:
import random from time import time class Proxy: def __init__(self, ip, success_rate=1.0, speed=1.0): self.ip = ip self.success_rate = success_rate # 0.0 to 1.0 self.speed = speed # lower is better self.last_used = 0 self.subnet = ip.split('.')[2] def calculate_weight(self, current_time, last_subnet): # Base weight weight = 1.0 # Success rate factor (higher success rate = higher weight) weight *= (0.5 + self.success_rate) # Speed factor (faster = higher weight) weight *= (2.0 - min(self.speed, 1.0)) # Time since last use factor (longer time = higher weight) time_factor = min((current_time - self.last_used) / 60.0, 5.0) # Cap at 5 minutes weight *= (0.5 + time_factor / 5.0) # Subnet diversity factor (different subnet = higher weight) if self.subnet == last_subnet: weight *= 0.5 return weight class ProxyRotator: def __init__(self, proxies): self.proxies = proxies self.last_subnet = None def get_proxy(self): current_time = time() weights = [p.calculate_weight(current_time, self.last_subnet) for p in self.proxies] # Select proxy based on weights selected_proxy = random.choices(self.proxies, weights=weights, k=1)[0] # Update proxy state selected_proxy.last_used = current_time self.last_subnet = selected_proxy.subnet return selected_proxy.ip
Time-Based Rotation and Cooldown Periods
Human users don't send requests at perfectly consistent intervals. Implementing variable timing and cooldown periods for each proxy helps mimic natural browsing patterns.
According to research, sophisticated anti-bot systems now analyze inter-request timing patterns, with randomized intervals reducing detection rates by 64% compared to fixed intervals.
Implement a time-based rotation strategy by:
- Enforcing minimum cooldown periods between uses of the same IP (30-60 seconds)
- Varying request intervals using a normal distribution (mimicking human patterns)
- Implementing longer cooldowns for IPs that encounter errors or CAPTCHAs
Building Your Own Proxy Rotation Infrastructure
If you're considering building your own rotating proxy infrastructure, here's a comprehensive framework:
Step 1: Acquire Proxy Sources
Begin by obtaining a diverse pool of proxies from reliable sources:
- Purchase datacenter or residential proxies from reputable providers
- Ensure geographic diversity (proxies from multiple countries/regions)
- Consider mixing proxy types for different scraping targets
For serious scraping operations, aim for at least 100 proxies distributed across various subnets.
Step 2: Implement a Proxy Manager
Create a proxy management system that handles:
- Storage and organization of your proxy pool
- Rotation logic based on the strategies outlined above
- Health monitoring and performance tracking
- Automatic blacklisting of failed or blocked proxies
Step 3: Integrate With Your Scraping Infrastructure
Connect your proxy manager to your web scraping tools:
# Python example using requests library import requests from proxy_manager import ProxyManager # Your custom manager proxy_manager = ProxyManager() def scrape_url(url): max_retries = 3 for attempt in range(max_retries): proxy = proxy_manager.get_proxy() try: response = requests.get( url, proxies={ 'http': f'http://{proxy}', 'https': f'http://{proxy}' }, timeout=10 ) if response.status_code == 200: proxy_manager.report_success(proxy) return response.text else: proxy_manager.report_failure(proxy, f"Status code: {response.status_code}") except Exception as e: proxy_manager.report_failure(proxy, str(e)) raise Exception(f"Failed to scrape {url} after {max_retries} attempts")
Step 4: Implement Monitoring and Analytics
Set up systems to track:
- Success rates by proxy and target domain
- Average response times
- Error rates and types
- Proxy usage patterns and rotation efficiency
These metrics will help you continuously optimize your proxy infrastructure.
Using Managed Rotating Proxy Services
Building your own rotating proxy infrastructure requires significant investment in development, maintenance, and proxy acquisition. For many organizations, using a managed proxy service is more cost-effective.
Benefits of Managed Rotating Proxy Services
- Access to larger proxy pools (millions of IPs vs. hundreds or thousands)
- Built-in intelligent rotation algorithms
- Automatic handling of proxy health and replacement
- Simplified integration through APIs or proxy endpoints
- Advanced features like browser fingerprinting and CAPTCHA solving
When selecting a provider, consider factors like:
- Proxy pool size and diversity
- Geographic coverage
- Pricing structure (per-request, per-GB, or subscription)
- Success rate guarantees
- Additional features like JavaScript rendering and CAPTCHA solving
Legal and Ethical Considerations
While using rotating proxies for web scraping is technically possible, it's crucial to understand the legal and ethical implications:
Legal Considerations
The legality of using rotating proxies for web scraping depends on various factors:
- Terms of Service compliance - Many websites explicitly prohibit scraping in their ToS
- Data usage rights - Just because data is public doesn't mean you can use it for any purpose
- Regional regulations - Laws like the CFAA in the US or the GDPR in Europe may apply
Ethical Considerations
Beyond legal concerns, consider the ethical implications:
- Server load - Aggressive scraping can impact website performance for legitimate users
- Respect for robots.txt - Following crawl directives shows respect for site owners
- Data privacy - Be careful when scraping and storing personal information
- Competitive fairness - Consider whether your scraping disadvantages competitors unfairly
Best Practices for Responsible Scraping
- Implement reasonable rate limiting (even with rotating proxies)
- Cache results to avoid unnecessary duplicate requests
- Scrape during off-peak hours when possible
- Consider official APIs as alternatives when available
- Be transparent about your data collection practices
The Future of Rotating Proxies and Web Scraping
The landscape of web scraping and rotating proxies continues to evolve rapidly:
Emerging Trends
- AI-powered anti-bot systems: Websites are increasingly using machine learning to identify and block scraping attempts based on behavioral patterns, not just IP addresses.
- Browser behavior emulation: Next-generation rotating proxy services now combine IP rotation with realistic browser fingerprinting and behavior simulation.
- Serverless scraping infrastructure: Cloud-based scraping solutions with built-in proxy rotation are becoming more popular for their scalability and ease of use.
- Ethical scraping frameworks: New tools are emerging that help developers scrape responsibly by enforcing best practices automatically.
Preparing for Future Challenges
To stay ahead in the web scraping arms race:
- Invest in diversified scraping approaches rather than relying solely on rotating proxies
- Monitor developments in anti-bot technologies to adapt your strategies accordingly
- Consider building relationships with data providers for more sustainable access
- Stay informed about evolving legal landscapes regarding web scraping
Field Notes: Community Perspectives on Rotating Proxies
Real-world experiences shared by engineers reveal a pragmatic approach to proxy selection that often contradicts theoretical discussions found in technical documentation. Many developers emphasize that decisions between rotating proxies, sticky sessions, or even using proxies at all should be driven primarily by practical results rather than best practices. This philosophy of "whatever works" appears consistently across various technical forums and discussions.
A recurring theme in community feedback highlights cost considerations as a primary factor. Several experienced developers recommend starting with the most economical solution—whether that's datacenter proxies or even no proxies—and only upgrading when actually encountering blocks. One senior engineer pointed out that bandwidth-based pricing models for rotating residential proxies can quickly become cost-prohibitive as scraping projects scale, suggesting that return on investment diminishes at higher volumes.
The community appears divided on proxy types based on specific use cases. While some developers consistently recommend rotating over sticky proxies due to cost efficiency, others emphasize that session-based scraping scenarios fundamentally require sticky IPs. Similarly, the datacenter versus residential proxy debate seems less about technical superiority and more about whether target websites actively block datacenter IPs. This nuanced, context-dependent approach reflects the complexity of modern web scraping challenges better than one-size-fits-all recommendations.
Conclusion: Building a Sustainable Web Scraping Strategy
Rotating proxies remain an essential component of effective web scraping in 2025, but they're just one piece of the puzzle. A truly resilient scraping operation combines:
- Sophisticated proxy rotation strategies
- Browser fingerprinting management
- Intelligent request timing
- Content parsing fallback mechanisms
- Respect for target website resources
Whether you build your own infrastructure or leverage managed services, understanding the principles behind effective proxy rotation will dramatically improve your scraping success rates while minimizing resource usage.
Remember that the most sustainable approach to web scraping balances technical effectiveness with legal compliance and ethical considerations. By implementing the strategies outlined in this guide, you'll be well-positioned to collect the data you need while navigating the complex technical and ethical landscape of web scraping at scale.
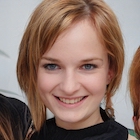