Web Scraping Best Practices in 2025: Expert Guide to Building Reliable & Ethical Data Collection Systems
Key Takeaways
- Build ethical scrapers by respecting robots.txt, implementing rate limiting, and following website terms of service
- Use a combination of rotating proxies, browser fingerprint management, and request throttling to avoid blocking
- Implement continuous data validation and error handling to ensure high-quality data collection
- Consider legal implications around copyright, GDPR, and terms of service before starting any scraping project
- Leverage modern tools like headless browsers and antidetect systems to handle dynamic content effectively
Introduction
Web scraping has become an essential tool for businesses and researchers to collect valuable data at scale. However, as websites implement increasingly sophisticated anti-bot measures, building reliable and ethical scrapers requires careful consideration of both technical and legal aspects. If you're wondering how to scrape websites without getting blocked, this guide will help you implement the right practices.
This comprehensive guide combines insights from industry experts with the latest best practices to help you build robust web scraping systems that respect website resources while maximizing success rates.
Technical Best Practices
1. Implement Smart Rate Limiting
The foundation of ethical scraping is respecting website resources. Modern rate limiting should go beyond simple delays:
- Implement adaptive delays based on server response times
- Use randomized intervals between requests (e.g., 2-5 seconds)
- Monitor server response codes and adjust accordingly
- Consider time-of-day patterns in website traffic
Example implementation in Python:
import time import random class AdaptiveRateLimiter: def __init__(self, base_delay=2): self.base_delay = base_delay self.last_request_time = 0 def wait(self, response_time=None): if response_time: # Adjust delay based on server response time delay = max(self.base_delay, response_time * 1.5) else: delay = self.base_delay # Add randomization delay += random.uniform(0, 2) # Ensure minimum wait time since last request time_since_last = time.time() - self.last_request_time if time_since_last < delay: time.sleep(delay - time_since_last) self.last_request_time = time.time()
2. Manage Browser Fingerprints
According to recent studies, over 30% of websites now implement some form of browser fingerprinting. Modern scraping systems need to manage multiple fingerprint aspects:
- Rotate User-Agent strings from an up-to-date list
- Randomize browser viewport sizes
- Manage WebGL fingerprints
- Handle canvas fingerprinting
3. Implement Robust Proxy Management
A reliable proxy infrastructure is crucial for large-scale scraping. For a detailed understanding of how to implement this effectively, check out our residential proxies implementation guide. Key considerations include:
- Use a mix of residential and datacenter proxies
- Implement intelligent proxy rotation based on failure rates
- Monitor proxy health and maintain backup pools
- Consider geographical distribution for better success rates
4. Handle Dynamic Content Effectively
Modern websites increasingly rely on JavaScript for content rendering. Effective approaches include:
- Use headless browsers like Playwright or Puppeteer for JavaScript-heavy sites
- Implement smart waiting strategies for dynamic content loading
- Consider using API endpoints when available
Example waiting strategy:
const waitForContent = async (page, selector, timeout = 5000) => { try { await page.waitForSelector(selector, { state: 'attached', timeout: timeout }); // Additional wait for content to stabilize await page.waitForFunction( (sel) => { const el = document.querySelector(sel); return el && el.getBoundingClientRect().height > 0; }, selector ); } catch (error) { console.error(`Timeout waiting for ${selector}`); throw error; } };
Data Quality and Validation
1. Implement Continuous Data Validation
Recent industry surveys show that up to 25% of scraped data can contain errors without proper validation. Implement checks at multiple levels:
- Schema validation for structured data
- Content-type checking
- Historical pattern comparison
- Automated anomaly detection
2. Handle Errors Gracefully
Proper error handling ensures reliable data collection:
- Implement retry mechanisms with exponential backoff
- Log detailed error information for debugging
- Set up monitoring and alerting for failure patterns
Legal and Ethical Considerations
1. Copyright and Terms of Service
Before starting any scraping project, consider:
- Review website terms of service
- Understand copyright implications for scraped content
- Document compliance measures
2. GDPR and Data Privacy
When handling personal data, ensure:
- Implement data minimization principles
- Establish clear data retention policies
- Document data processing purposes
Tools and Resources
Popular Scraping Frameworks
Framework | Best For | Key Features |
---|---|---|
Scrapy | Large-scale projects | Built-in middleware, pipeline support |
Playwright | Dynamic websites | Modern browser automation, network interception |
Selenium | Complex interactions | Broad browser support, mature ecosystem |
Case Study: Building a Resilient E-commerce Scraper
A recent project required scraping product data from major e-commerce platforms. Key challenges and solutions included:
- Challenge: Dynamic pricing updates
- Solution: Implemented WebSocket monitoring
- Result: 99.8% accuracy in price tracking
- Challenge: Anti-bot detection
- Solution: Browser fingerprint randomization
- Result: Reduced blocking rate from 30% to 2%
Future Trends in Web Scraping
Looking ahead to 2025-2026, several trends are shaping the future of web scraping:
- AI-powered anti-bot systems requiring more sophisticated evasion techniques
- Increased use of WebSocket and GraphQL APIs
- Stricter data privacy regulations affecting scraping practices
Field Notes: Developer Experiences
Technical discussions across various platforms reveal a nuanced perspective on web scraping approaches, with developers emphasizing the importance of thoughtful planning and incremental development. To avoid common pitfalls, review these common web scraping mistakes that beginners make. Experienced practitioners often advise newcomers to start with the fundamentals - understanding HTML structure, mastering developer tools (particularly the F12 inspector), and beginning with simpler parsing libraries like Beautiful Soup before moving to more complex solutions.
A recurring theme in developer forums is the debate between frontend scraping versus API utilization. While many developers advocate for using official APIs when available, noting their reliability and cleaner data structure, others point out that paid API services may not be cost-effective for smaller projects. This has led to pragmatic hybrid approaches where teams use APIs for critical data collection while supplementing with frontend scraping for additional data points.
Real-world implementations have revealed several practical insights about scraping dynamic content. Developers report success with a staged approach: starting with static HTML parsing, then incorporating JavaScript rendering capabilities through tools like Playwright or Selenium when necessary. However, they caution that JavaScript-heavy sites require careful consideration of execution timing and asynchronous operations, which can significantly impact scraping reliability.
The community also emphasizes the importance of defensive programming in scraping projects. Experienced developers recommend implementing robust error handling, caching mechanisms for development, and thorough testing with saved copies of pages before hitting live sites. This approach not only respects website resources but also accelerates the development process by reducing unnecessary requests during the testing phase.
Conclusion
Successful web scraping requires a balanced approach that combines technical expertise with ethical considerations. If you're looking to implement large-scale data collection, explore our guide on proxy servers for scraping data. By following these best practices and staying updated with the latest trends, you can build reliable scraping systems that respect both website resources and user privacy while delivering high-quality data.
For more detailed information on specific topics, consider exploring these resources:
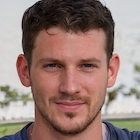